c++实现完整的单向链表
时间: 2023-12-26 14:24:04 浏览: 27
在 C 语言中,我们可以通过定义一个结构体来实现完整的单向链表。
首先,我们定义一个表示链表节点的结构体,包含一个数据项和一个指向下一个节点的指针:
```c
struct Node {
int data;
struct Node* next;
};
```
然后,我们可以编写一些函数来操作链表。下面是一些常用的操作函数:
1. 创建链表:创建一个空链表,并返回指向链表头节点的指针。
```c
struct Node* createList() {
return NULL;
}
```
2. 插入节点:在链表的特定位置插入一个新节点。
```c
void insertNode(struct Node** head, int data, int position) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = NULL;
if (position == 0) {
newNode->next = *head;
*head = newNode;
} else {
struct Node* current = *head;
int i;
for (i = 0; i < position - 1 && current != NULL; i++) {
current = current->next;
}
if (current != NULL) {
newNode->next = current->next;
current->next = newNode;
}
}
}
```
3. 删除节点:删除链表中特定位置的节点。
```c
void deleteNode(struct Node** head, int position) {
if (*head == NULL) {
return;
}
struct Node* temp = *head;
if (position == 0) {
*head = temp->next;
free(temp);
return;
}
int i;
for (i = 0; temp != NULL && i < position - 1; i++) {
temp = temp->next;
}
if (temp == NULL || temp->next == NULL) {
return;
}
struct Node* nextNode = temp->next->next;
free(temp->next);
temp->next = nextNode;
}
```
4. 打印链表:遍历链表并打印每个节点的数据。
```c
void printList(struct Node* head) {
struct Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
```
这些函数可以帮助我们创建、插入、删除和打印链表。以下是示例使用代码:
```c
int main() {
struct Node* myList = createList();
insertNode(&myList, 1, 0);
insertNode(&myList, 2, 1);
insertNode(&myList, 3, 2);
printList(myList); // 输出:1 2 3
deleteNode(&myList, 1);
printList(myList); // 输出:1 3
return 0;
}
```
相关推荐
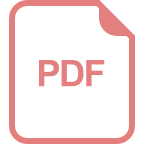














