用c++写一段完整的代码,要求实现单向链表删除节点,不要出现语法错误
时间: 2023-06-01 22:02:38 浏览: 45
以下是一段 C 代码,实现了单向链表的删除节点功能:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
struct Node* head = NULL;
void insert(int data) {
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
new_node->data = data;
new_node->next = head;
head = new_node;
}
void delete(int key) {
struct Node* temp = head;
struct Node* prev = NULL;
if (temp != NULL && temp->data == key) {
head = temp->next;
free(temp);
return;
}
while (temp != NULL && temp->data != key) {
prev = temp;
temp = temp->next;
}
if (temp == NULL) {
return;
}
prev->next = temp->next;
free(temp);
}
void print() {
struct Node* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
int main() {
insert(5);
insert(4);
insert(3);
insert(2);
insert(1);
printf("List before deletion: ");
print();
delete(3);
printf("List after deletion: ");
print();
return 0;
}
```
以上代码实现了单向链表的删除节点功能,通过 insert 函数向链表中插入数据,delete 函数删除链表中指定的节点,print 函数打印链表中的所有数据。在 main 函数中插入了 5 个数据,然后删除了值为 3 的节点,最后输出链表中的数据。
相关推荐
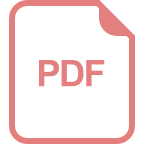
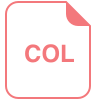
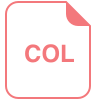
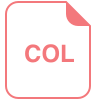
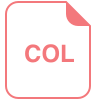
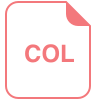









