使用C++实现简单的数据结构:链表与二叉树
发布时间: 2023-12-30 11:47:47 阅读量: 10 订阅数: 12 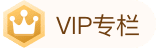
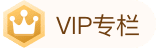
# 1. 引言
## 1.1 介绍数据结构的重要性
数据结构是计算机科学中的重要内容,它是研究数据组织、存储和管理的一门学科。在实际的软件开发过程中,有效地选择和使用合适的数据结构至关重要。不同的数据结构适用于不同的应用场景,能够提高算法的效率、节省存储空间,并简化程序的设计和实现。
## 1.2 简要介绍链表和二叉树
链表和二叉树是常见的数据结构,它们在实际的软件开发中经常被使用到。链表是一种线性数据结构,由一系列的节点组成,每个节点包含数据和指向下一个节点的指针。链表可以方便地插入和删除节点,但查找节点的效率相对较低。而二叉树是一种树形结构,每个节点最多有两个子节点,左子节点和右子节点。二叉树可以高效地进行查找、插入和删除操作,特别适合用于排序和搜索问题。
接下来,我们将深入介绍链表和二叉树的实现方法和应用案例。
### 2. 链表的实现
链表是一种常见的线性数据结构,它由一系列节点组成,每个节点包含数据和指向下一个节点的指针。链表相比于数组,具有插入和删除操作更为灵活的特点,但查找操作的效率较低。
#### 2.1 链表的基本概念
链表由节点组成,每个节点包含两部分数据:存储的元素值和指向下一节点的指针。链表的第一个节点称为头节点,最后一个节点称为尾节点,尾节点的指针指向空值(NULL)。
#### 2.2 使用C语言定义链表结构体
```c
#include <stdio.h>
#include <stdlib.h>
// 链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建一个新节点
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 定义链表结构体
typedef struct LinkedList {
Node* head;
int size;
} LinkedList;
// 初始化链表
void initLinkedList(LinkedList* list) {
list->head = NULL;
list->size = 0;
}
// 其他链表操作函数(插入、删除、遍历等)省略
```
#### 2.3 实现链表的插入和删除操作
```c
// 在链表末尾插入节点
void insertNodeAtEnd(LinkedList* list, int data) {
Node* newNode = createNode(data);
if (list->head == NULL) {
list->head = newNode;
} else {
Node* current = list->head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
list->size++;
}
// 从链表中删除指定数值的节点
void deleteNodeWithValue(LinkedList* list, int data) {
Node* current = list->head;
Node* prev = NULL;
while (current != NULL && current->data != data) {
prev = current;
current = current->next;
}
if (current != NULL) {
if (prev != NULL) {
prev->next = current->next;
} else {
list->head = current->next;
}
free(current);
list->size--;
}
}
```
#### 2.4 链表的遍历和查找
```c
// 遍历链表并打印节点元素
void printLinkedList(LinkedList* list) {
Node* current = list->head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
}
// 在链表中查找指定数值的节点
Node* searchNodeWithValue(LinkedList* list, int data) {
Node* current = list->head;
while (current != NULL && current->data != data) {
current = current->next;
}
return current;
}
```
本节介绍了链表的基本概念,并使用C语言定义了链表结构体,以及实现了链表的插入、删除、遍历和查找操作。接下来将通过应用案例展示链表的实际应用。
### 3. 链表的应用案例
链表作为一种基本的数据结构,在实际的软件开发中有着丰富的应用场景,下面我们将介绍两个链表的应用案例。
#### 3.1 实现一个简单的图书管理系统
假设我们需要实现一个简单的图书管理系统,其中需要对图书信息进行增删改查操作。我们可以使用链表来存储图书信息,每个节点表示一本图书,包含图书的名称、作者、ISBN号等信息。我们定义一个图书类 Book,然后创建一个链表来存储图书信息。
```java
class Book {
String title;
String author;
String ISBN;
Book next;
public Book(String title, String author, String ISBN) {
this.title = title;
this.author = author;
this.ISBN = ISBN;
this.next = null;
}
}
class BookList {
Book head;
public BookList() {
this.head = null;
}
// 插入操作
public void insert(Book newBook) {
if (head == null) {
head = newBook;
} else {
newBook.next = head;
head = newBook;
}
}
// 删除操作
public void delete(String ISBN) {
Book current = head;
Book previous = null;
while (current != null && !current.ISBN.equals(ISBN)) {
previous = current;
current = current.next;
}
```
0
0
相关推荐
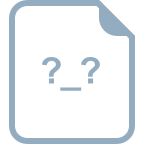
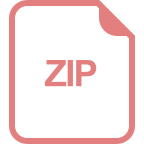
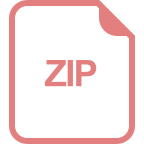





