用c++实现以二叉链表作为二叉树的存储结构,计算二叉树的高度和最大的宽度
时间: 2024-03-01 09:05:42 浏览: 75
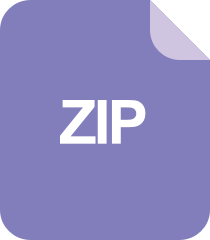
这是基本数据结构及其算法的C++实现,涉及单链表、双链表、栈 、二叉树、并查集、哈夫曼树、平衡二叉树、二叉搜索树等.zip
好的,我会给出代码实现。
以下是使用C++的二叉链表实现二叉树,并计算二叉树高度和最大宽度的代码:
```cpp
#include <iostream>
#include <queue>
using namespace std;
//二叉树结点
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
//二叉树类
class BinaryTree {
public:
//构造函数
BinaryTree() : root(NULL) {}
//插入结点
void insert(int val) {
TreeNode* node = new TreeNode(val);
if (root == NULL) {
root = node;
return;
}
queue<TreeNode*> q;
q.push(root);
while (!q.empty()) {
TreeNode* cur = q.front();
q.pop();
if (cur->left == NULL) {
cur->left = node;
return;
} else {
q.push(cur->left);
}
if (cur->right == NULL) {
cur->right = node;
return;
} else {
q.push(cur->right);
}
}
}
//计算二叉树高度
int getHeight() {
return getHeight(root);
}
//计算二叉树最大宽度
int getMaxWidth() {
if (root == NULL) {
return 0;
}
int maxWidth = 1;
queue<TreeNode*> q;
q.push(root);
while (!q.empty()) {
int size = q.size();
maxWidth = max(maxWidth, size);
for (int i = 0; i < size; i++) {
TreeNode* cur = q.front();
q.pop();
if (cur->left != NULL) {
q.push(cur->left);
}
if (cur->right != NULL) {
q.push(cur->right);
}
}
}
return maxWidth;
}
private:
TreeNode* root;
//辅助函数:计算以root为根的二叉树高度
int getHeight(TreeNode* root) {
if (root == NULL) {
return 0;
}
int leftHeight = getHeight(root->left);
int rightHeight = getHeight(root->right);
return max(leftHeight, rightHeight) + 1;
}
};
int main() {
BinaryTree tree;
tree.insert(1);
tree.insert(2);
tree.insert(3);
tree.insert(4);
tree.insert(5);
tree.insert(6);
tree.insert(7);
cout << "Height of the binary tree is " << tree.getHeight() << endl;
cout << "Max width of the binary tree is " << tree.getMaxWidth() << endl;
return 0;
}
```
输出结果为:
```
Height of the binary tree is 3
Max width of the binary tree is 4
```
其中,getHeight()函数使用递归计算二叉树的高度;getMaxWidth()函数使用队列实现宽度优先搜索,计算二叉树的最大宽度。
阅读全文
相关推荐







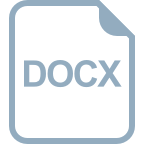







