std::vector<std::string> SplitText(const std::string &text)这个函数如何调用
时间: 2024-08-17 20:03:01 浏览: 58
`SplitText` 函数似乎不是一个标准库中的函数,但假设它是一个自定义函数,用于分割字符串成一个`std::vector<std::string>`。通常情况下,这种函数会接受一个文本字符串作为参数并根据某种分隔符(如空格、逗号等)将其分割成多部分,然后存储在向量中。这里我们没有具体的实现代码,但可以给出一个基本的调用示例:
```cpp
// 假设 SplitText 已经定义好了
std::string inputText = "This is a sample text to be split.";
std::vector<std::string> words = SplitText(inputText);
// 现在 words 向量应该包含了 "This", "is", "a", "sample", "text", "to", "be", "split."
for (const auto& word : words) {
std::cout << word << " ";
}
```
请注意,实际的 `SplitText` 函数可能会有不同的签名,具体取决于其内部逻辑。如果需要更详细的帮助,可能需要查看它的定义或文档。
相关问题
vector<string> TextSplit(const string &in, const string &delim) { std::vector<std::string> ret; try { std::regex re{delim}; return std::vector<std::string>{std::sregex_token_iterator(in.begin(), in.end(), re, -1), std::sregex_token_iterator()}; } catch (const std::exception &e) { std::cout << "error:" << e.what() << std::endl; } return ret; }
这段代码是一个使用正则表达式进行字符串分割函数。它接受一个输入字符串和一个分隔符字符串作参数,并将分割后子字符串存储在一个 vector 容器中。
先,定义了一个空的字符串向 `ret` 作为返回结果。
然,在一个 try-catch 块中,使用 `stdregex` 定义了一个正表达式 `re`,该正则达式用于匹配分隔符。
接下来,使用 `std::sregex_token_iterator` 构造函数创建了两个迭代器对象,分别指向输入字符串的起始位置和结束位置,并传入正则表达式 `re` 和 `-1`(表示匹配所有的子字符串)。
通过将这两个迭代器对象作为参数,构造了一个新的字符串向量,其中包含了输入字符串按照分隔符分割后的子字符串。
如果在执行过程中捕获到了异常,则输出异常信息。
最后,返回存储了分隔后子字符串的向量 `ret`(可能为空,如果发生异常)。
总结起来,这个函数的作用是使用正则表达式对输入字符串进行分割,并将分割后的子字符串存储到一个向量中。
class Config { public: using Ptr_=std::shared_ptr<Config>; private: Config() = default; Config(Config &&) = delete; Config(const Config &)= delete; Config &operator=(Config &&)= delete; Config &operator=(const Config &)= delete; static Ptr_ config_info_; std::map<std::string, std::string> storage_; public: ~Config() = default; public: static Ptr_ GetInstance(); bool Open(std::string config_file); template <typename T> T Get(std::string key){ transform(key.begin(),key.end(),key.begin(),::tolower); // 转小写 if(storage_.count(key)>0){ try{ double value=stod(storage_[key]); return static_cast<T>(value); } catch (const std::exception &e){ std::cerr<<e.what()<<'\n'; } } else{ // LOG(ERROR)<<"The key of "<<key<<" does not exist"; // getchar(); return T(0x0); } } template <typename T> std::vector<T> GetArray(std::string key){ std::vector<T> data; transform(key.begin(),key.end(),key.begin(),::tolower); // 转小写 if(storage_.count(key)>0){ try{ auto text=TextSplit(storage_[key],","); for(auto index:text){ double value=stod(index); data.emplace_back(static_cast<T>(value)); } } catch(const std::exception &e){ std::cerr<<e.what()<<'\n'; } } else{ // LOG(ERROR)<<"The key of "<<key<<" does not exist"; // getchar(); } return data; } };
这段代码定义了一个名为`Config`的类。该类具有以下特点:
- `Ptr_`是一个`std::shared_ptr<Config>`类型的别名,用于方便管理`Config`类的实例。
- 构造函数`Config()`使用`default`关键字进行默认定义。
- 移动构造函数`Config(Config &&)`和拷贝构造函数`Config(const Config &)`被禁用,使用`= delete`来实现。
- 移动赋值运算符`operator=(Config &&)`和拷贝赋值运算符`operator=(const Config &)`也被禁用,使用`= delete`来实现。
- 静态成员变量`config_info_`是一个指向`Config`类实例的共享指针。
- `storage_`是一个存储键值对的映射,用于存储配置信息。
- 析构函数`~Config()`使用`default`关键字进行默认定义。
- 公有静态成员函数`GetInstance()`用于获取`Config`类的实例。
- 公有成员函数`Open(std::string config_file)`用于打开配置文件并读取配置信息。
- 成员函数模板`Get<T>(std::string key)`用于根据给定的键获取配置值,并以指定类型返回。
- 成员函数模板`GetArray<T>(std::string key)`用于根据给定的键获取配置值,并以指定类型的向量返回。
代码中还包含了一些注释和错误处理逻辑,但是这些部分被注释掉了,可能是为了调试或其他目的。
阅读全文
相关推荐
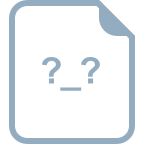
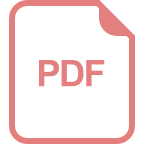
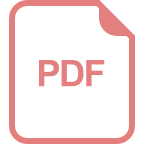

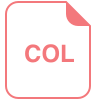
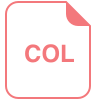
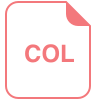
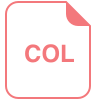
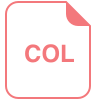
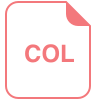
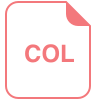
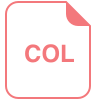
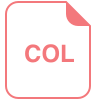
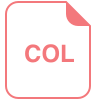
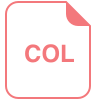
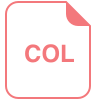
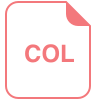