对‘split(std::__cxx11::string&, const char [2])’的调用没有匹配的函数
时间: 2024-03-17 07:39:42 浏览: 196
这个错误的原因可能是你传递给 `split()` 函数的第二个参数类型不匹配,因为 C++ 中的字符串字面量的类型是 `const char[]`,而不是 `const char*`。你可以将函数声明中的分隔符参数类型改为 `const char*` 或者将字符串字面量改为字符。下面是两种解决方法的示例:
方法一:将分隔符参数类型改为 `const char*`,示例代码如下:
```c++
#include <iostream>
#include <vector>
#include <string>
std::vector<std::string> split(std::string& s, const char* delimiter) {
std::vector<std::string> tokens;
size_t pos = 0;
while ((pos = s.find(delimiter)) != std::string::npos) {
std::string token = s.substr(0, pos);
tokens.push_back(token);
s.erase(0, pos + strlen(delimiter));
}
tokens.push_back(s);
return tokens;
}
int main() {
std::string text = "Hello,world!";
std::vector<std::string> words = split(text, ",");
for (const auto& word : words) {
std::cout << word << std::endl;
}
return 0;
}
```
在这个示例中,我们将 `split()` 函数的第二个参数类型从 `const char[]` 改为 `const char*`,并且使用 `strlen()` 函数获取分隔符的长度。这样就可以正确地分割字符串了。
方法二:将字符串字面量改为字符,示例代码如下:
```c++
#include <iostream>
#include <vector>
#include <string>
std::vector<std::string> split(std::string& s, char delimiter) {
std::vector<std::string> tokens;
size_t pos = 0;
while ((pos = s.find(delimiter)) != std::string::npos) {
std::string token = s.substr(0, pos);
tokens.push_back(token);
s.erase(0, pos + 1);
}
tokens.push_back(s);
return tokens;
}
int main() {
std::string text = "Hello,world!";
std::vector<std::string> words = split(text, ',');
for (const auto& word : words) {
std::cout << word << std::endl;
}
return 0;
}
```
在这个示例中,我们将 `split()` 函数的第二个参数改为字符。这样也可以正确地分割字符串。
阅读全文
相关推荐
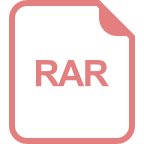
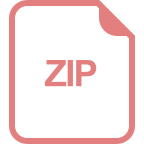
















