用OSGEarth实现导弹仿真发射的代码
时间: 2024-06-09 07:09:04 浏览: 190
由于导弹仿真发射涉及到很多细节和复杂的物理模拟,这里简单介绍基于OSGEarth的可视化部分的实现。
首先,我们需要使用OSGEarth创建一个地球场景,并将其渲染到窗口中。可以参考OSGEarth官方文档进行安装和使用。
接下来,我们需要添加一个导弹模型,可以使用OSG的Node模型来实现。这里简单介绍如何添加一个模型:
```c++
// 创建一个模型节点
osg::ref_ptr<osg::Node> missileModel = osgDB::readNodeFile("missile.ive");
// 设置模型的位置和旋转
osg::MatrixTransform* missileTransform = new osg::MatrixTransform;
missileTransform->addChild(missileModel);
missileTransform->setMatrix(osg::Matrix::translate(0, 0, 0) *
osg::Matrix::rotate(osg::DegreesToRadians(90.0), 1, 0, 0));
// 将模型添加到场景中
osg::ref_ptr<osg::Group> root = new osg::Group;
root->addChild(missileTransform);
// 将场景设置为OSGEarth的地球场景
osgEarth::MapNode* mapNode = osgEarth::MapNode::findMapNode(root);
if (mapNode)
{
osg::ref_ptr<osgEarth::Util::EarthManipulator> manipulator =
new osgEarth::Util::EarthManipulator;
manipulator->setMapNode(mapNode);
viewer.setCameraManipulator(manipulator);
viewer.setSceneData(mapNode);
}
```
接下来,我们需要实现导弹的发射过程。这里简单介绍如何使用OSG的动画系统实现导弹的运动:
```c++
osg::ref_ptr<osg::AnimationPath> path = new osg::AnimationPath;
path->setLoopMode(osg::AnimationPath::NO_LOOPING);
// 添加关键帧
path->insert(0.0, osg::AnimationPath::ControlPoint(
osg::Vec3d(0, 0, 0), osg::Quat(0, osg::Vec3d(0, 0, 1))));
path->insert(5.0, osg::AnimationPath::ControlPoint(
osg::Vec3d(0, 0, 10000), osg::Quat(osg::DegreesToRadians(90.0), osg::Vec3d(1, 0, 0))));
path->insert(10.0, osg::AnimationPath::ControlPoint(
osg::Vec3d(0, 0, 20000), osg::Quat(osg::DegreesToRadians(180.0), osg::Vec3d(0, 1, 0))));
path->insert(15.0, osg::AnimationPath::ControlPoint(
osg::Vec3d(0, 0, 30000), osg::Quat(osg::DegreesToRadians(270.0), osg::Vec3d(0, 0, 1))));
path->insert(20.0, osg::AnimationPath::ControlPoint(
osg::Vec3d(0, 0, 40000), osg::Quat(osg::DegreesToRadians(360.0), osg::Vec3d(1, 1, 0))));
// 创建动画路径回调
osg::ref_ptr<osg::AnimationPathCallback> pathCallback =
new osg::AnimationPathCallback(path.get(), 0.0, 1.0);
missileTransform->setUpdateCallback(pathCallback.get());
```
最后,我们需要添加一些场景元素来模拟导弹发射,例如发射井、地面等等。这里可以使用OSG的Geometry模块来实现。
完整的代码实现可以参考OSGEarth官方文档和OSG官方文档。
阅读全文
相关推荐
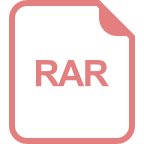













