c++处理protobuf
时间: 2023-08-19 15:05:24 浏览: 60
处理protobuf的C++代码可以通过使用protocol buffers编译器来实现。首先,你需要安装protocol buffers编译器,并确保它已经添加到系统的环境变量中。然后,你可以使用以下命令来调用编译器生成C++代码:
```
protoc --cpp_out=DST_DIR path/to/file.proto
```
其中,`DST_DIR`是指定生成的C++代码的目录,`path/to/file.proto`是你的.proto文件的路径。编译器将根据.proto文件中定义的消息类型生成相应的C++代码。
在生成的C++代码中,你可以使用`SerializeToString`函数将消息对象序列化为字符串,使用`ParseFromString`函数将字符串反序列化为消息对象。你可以根据需要设置消息对象的字段值,并通过访问器函数获取字段值。
以下是一个处理protobuf的C++代码的示例:
```cpp
#include <iostream>
#include <fstream>
#include "addressbook.pb.h"
using namespace std;
int main(int argc, char** argv) {
// 创建一个消息对象
IM::Account account1;
account1.set_id(1);
account1.set_name("windsun");
account1.set_password("123456");
// 将消息对象序列化为字符串
string serializeToStr;
account1.SerializeToString(&serializeToStr);
cout << "序列化后的字节:" << serializeToStr << endl;
// 创建另一个消息对象,并从字符串中反序列化
IM::Account account2;
if (!account2.ParseFromString(serializeToStr)) {
cerr << "failed to parse student." << endl;
return -1;
}
// 输出反序列化后的字段值
cout << "反序列化:" << endl;
cout << account2.id() << endl;
cout << account2.name() << endl;
cout << account2.password() << endl;
// 关闭protobuf库
google::protobuf::ShutdownProtobufLibrary();
return 0;
}
```
在这个示例中,我们首先创建了一个`IM::Account`消息对象,并设置了其字段值。然后,我们使用`SerializeToString`函数将其序列化为字符串,并输出序列化后的字节。接下来,我们创建了另一个`IM::Account`消息对象,并使用`ParseFromString`函数从字符串中反序列化。最后,我们输出反序列化后的字段值。
请注意,你需要根据你的.proto文件中定义的消息类型进行相应的修改。另外,你还需要包含相应的头文件和链接protocol buffers库。
相关推荐
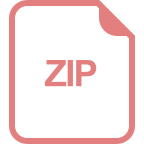
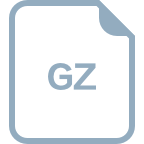














