void *thread(void *arg) 20 { 21 char *ptr = NULL; 22 23 /*建立线程清理程序*/ 24 printf("this is new thread\n"); 25 ptr = (char*)malloc(100); 26 pthread_cleanup_push(cleanup_func1, (void*)(ptr)); 27 pthread_cleanup_push(cleanup_func2, NULL); 28 bzero(ptr, 100); 29 strcpy(ptr, "memory from malloc"); 30 /*注意push与pop必须配对使用,即使pop执行不到*/ 31 sleep(3); 32 printf("before pop\n"); 33 pthread_cleanup_pop(1); 34 printf("before pop\n"); 35 pthread_cleanup_pop(1); 36 return NULL; 37 }
时间: 2023-05-12 09:05:36 浏览: 82
这是一个线程函数,使用了pthread库。在第25行使用了malloc函数分配了100字节的内存,第26行和第27行使用了pthread_cleanup_push函数注册了两个线程清理程序,第33行和第35行使用了pthread_cleanup_pop函数弹出了这两个线程清理程序。在第28行使用了bzero函数将分配的内存清零,在第29行使用了strcpy函数将字符串"memory from malloc"复制到了分配的内存中。在第31行使用了sleep函数暂停了3秒,然后在第32行和第34行分别输出了"before pop"。最后在第36行返回了NULL。
相关问题
linux void*thread(void*arg)
这是一个Linux下创建线程的函数,其函数原型为:
```c
int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine) (void *), void *arg);
```
该函数的参数解释如下:
- `thread`:指向线程标识符的指针,用于存储新创建线程的标识符。
- `attr`:线程属性,通常设为 NULL。
- `start_routine`:指向线程函数的指针,新创建的线程将从该函数的起始地址开始执行。
- `arg`:传递给线程函数的参数。
函数返回值为 0 表示线程创建成功,否则返回错误码。
需要注意的是,线程函数必须返回 `void*` 类型的指针,并且参数也是 `void*` 类型的指针。在线程函数内部,可以通过强制类型转换将参数还原为其实际类型。例如:
```c
void *my_thread_func(void *arg) {
int my_arg = *((int *) arg);
// ...
return NULL;
}
```
void* Run( void *arg)
函数 `Run` 是一个线程函数,它接受一个 `void*` 类型的参数 `arg`,并返回一个 `void*` 类型的指针。
在这个函数中,你可以执行一些与线程相关的操作。通常情况下,你会将需要在线程中执行的代码放在这个函数中,并将其作为线程的入口点。
函数签名 `void* Run( void *arg)` 中的 `void*` 表示返回一个指向任意类型的指针,`arg` 参数也是一个指向任意类型的指针。这样设计的目的是为了使函数更加通用,可以适应不同类型的参数和返回值。
你可以根据具体的需求,在 `Run` 函数中进行线程的初始化、执行任务、释放资源等操作。
相关推荐
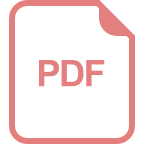
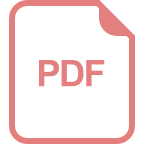
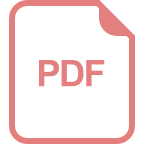












