用jupyter实现一个图片识别界面,算法模型为自定义,类型为pth
时间: 2023-06-14 15:08:18 浏览: 42
首先,我们需要创建一个 Jupyter Notebook,并安装必要的库。
1. 安装 PyTorch 库:
```python
!pip install torch
```
2. 安装 torchvision 库:
```python
!pip install torchvision
```
3. 安装 Pillow 库:
```python
!pip install Pillow
```
接下来,我们需要下载并加载自定义的模型。假设我们的模型文件为 `model.pth`,我们可以使用以下代码加载模型:
```python
import torch
# 加载模型
model = torch.load('model.pth')
```
在加载模型之后,我们可以定义一个函数来使用模型进行图像分类。假设我们的模型是一个分类器,它可以将图像分为两个类别:狗和猫。
```python
import torch
from PIL import Image
import torchvision.transforms as transforms
# 加载模型
model = torch.load('model.pth')
# 定义标签
labels = ['cat', 'dog']
# 定义预处理函数
preprocess = transforms.Compose([
transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize(
mean=[0.485, 0.456, 0.406],
std=[0.229, 0.224, 0.225]
)
])
def predict_image(image_path):
# 加载图像
image = Image.open(image_path)
# 预处理图像
image_tensor = preprocess(image)
# 添加批次维度
image_tensor = image_tensor.unsqueeze(0)
# 将图像传递给模型
output = model(image_tensor)
# 获取预测结果
_, predicted = torch.max(output, 1)
# 返回预测结果
return labels[predicted[0]]
```
现在,我们可以定义一个简单的界面来交互式地使用我们的模型进行图像分类。假设我们使用 `ipywidgets` 库来创建界面。
```python
import ipywidgets as widgets
from IPython.display import display
# 创建文件选择器
file_selector = widgets.FileUpload()
# 创建输出框
output = widgets.Output()
# 创建预测函数
def predict_image_button_clicked(_):
# 获取文件路径
filename = list(file_selector.value.keys())[0]
# 预测图像
predicted_label = predict_image(filename)
# 在输出框中显示预测结果
with output:
print('Predicted label:', predicted_label)
# 创建预测按钮
predict_image_button = widgets.Button(description='Predict image')
# 将预测函数与预测按钮相关联
predict_image_button.on_click(predict_image_button_clicked)
# 显示文件选择器、预测按钮和输出框
display(file_selector)
display(predict_image_button)
display(output)
```
现在,我们的界面已经准备好了。我们可以通过上传图像并单击预测按钮来使用我们的模型进行图像分类。
相关推荐
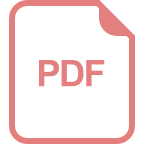
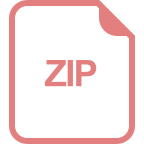





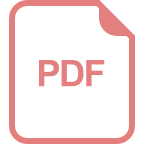
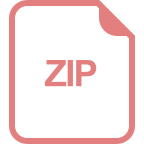
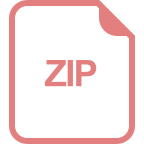
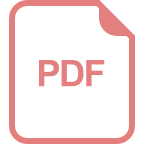
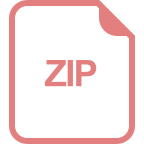
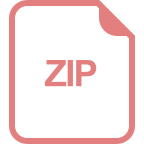