#include <stdio.h>#include <stdlib.h>#include <iostream>#include <string>#include <vector>#include <cassert>
时间: 2024-08-15 16:03:17 浏览: 61
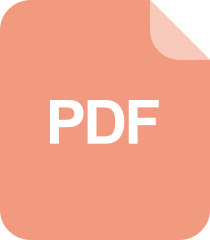
C标准库函数集

C++中也有多种方式来找到数组、向量或其他容器中的最大值。这里是一些示例:
1. 对整数数组使用`std::max_element`[^4]:
```cpp
#include <algorithm>
int main() {
int arr[] = {5, 10, 15, 20, 25};
auto it = std::max_element(arr, arr + sizeof(arr) / sizeof(int));
std::cout << "Max value in array: " << *it << '\n'; // 输出最大值
}
```
2. 对向量使用`*std::max_element`[^5]:
```cpp
#include <vector>
std::vector<int> vec = {456, 700, 200};
auto max_val = *std::max_element(vec.begin(), vec.end());
std::cout << "Max value in vector: " << max_val << '\n';
```
3. 对字符串比较通常是通过字符逐个比较,但可以借助`std::lexicographical_compare`[^6]实现:
```cpp
#include <string>
std::string str1 = "hello";
std::string str2 = "world";
if (std::lexicographical_compare(str1.begin(), str1.end(), str2.begin(), str2.end())) {
std::cout << "Max string lexicographically: " << str1 << '\n';
} else {
std::cout << "Max string lexicographically: " << str2 << '\n';
}
```
请注意,对于字符串,这里的"最大"是指字典序上的最大。
阅读全文
相关推荐
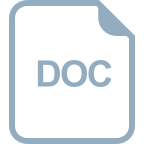
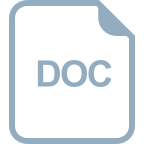









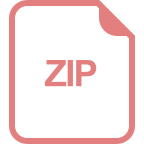
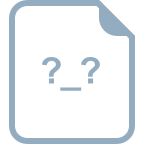
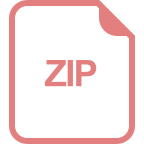
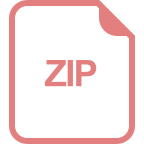
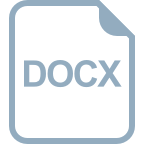
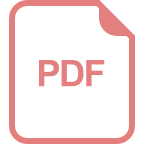
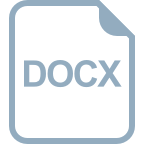