用pycharm俄罗斯方块
时间: 2024-01-26 09:14:03 浏览: 39
在PyCharm中实现俄罗斯方块游戏可以按照以下步骤进行:
1. 创建一个新的PyCharm项目。
2. 在项目中创建一个Python文件,命名为"tetris.py"。
3. 导入所需的模块,包括pygame和random。
4. 初始化pygame并设置游戏窗口的大小。
5. 创建一个方块类,包括方块的形状、颜色和位置等属性,以及方块的移动和旋转方法。
6. 创建一个游戏类,包括游戏的初始化、更新和绘制方法,以及处理用户输入的方法。
7. 在游戏类中创建一个方块实例,并在游戏循环中更新和绘制方块。
8. 处理方块的移动、旋转和停靠等操作,并检测是否有行被填满并消除。
9. 在游戏结束时显示得分并退出游戏。
下面是一个简单的示例代码:
```python
import pygame
import random
# 游戏窗口大小
WINDOW_WIDTH = 800
WINDOW_HEIGHT = 600
# 方块大小
BLOCK_SIZE = 30
# 方块颜色
COLORS = [(0, 0, 0), (255, 0, 0), (0, 255, 0), (0, 0, 255), (255, 255, 0), (255, 0, 255), (0, 255, 255), (255, 255, 255)]
# 方块形状
SHAPES = [
[[1, 1, 1, 1]],
[[1, 1], [1, 1]],
[[1, 1, 0], [0, 1, 1]],
[[0, 1, 1], [1, 1, 0]],
[[1, 1, 1], [0, 1, 0]],
[[1, 1, 1], [1, 0, 0]],
[[1, 1, 1], [0, 0, 1]]
]
class Block:
def __init__(self):
self.shape = random.choice(SHAPES)
self.color = random.choice(COLORS)
self.x = WINDOW_WIDTH // 2 - BLOCK_SIZE
self.y = 0
def move_down(self):
self.y += BLOCK_SIZE
def move_left(self):
self.x -= BLOCK_SIZE
def move_right(self):
self.x += BLOCK_SIZE
def rotate(self):
self.shape = list(zip(*reversed(self.shape)))
def draw(self, surface):
for i in range(len(self.shape)):
for j in range(len(self.shape[i])):
if self.shape[i][j] == 1:
pygame.draw.rect(surface, self.color, (self.x + j * BLOCK_SIZE, self.y + i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
class Game:
def __init__(self):
pygame.init()
self.surface = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption("Tetris")
self.clock = pygame.time.Clock()
self.block = Block()
self.grid = [[0] * (WINDOW_WIDTH // BLOCK_SIZE) for _ in range(WINDOW_HEIGHT // BLOCK_SIZE)]
self.score = 0
def update(self):
self.block.move_down()
if self.collide():
self.block.move_up()
self.freeze()
self.clear_lines()
self.block = Block()
def collide(self):
for i in range(len(self.block.shape)):
for j in range(len(self.block.shape[i])):
if self.block.shape[i][j] == 1:
if self.block.y + i * BLOCK_SIZE >= WINDOW_HEIGHT or self.grid[(self.block.y + i * BLOCK_SIZE) // BLOCK_SIZE][(self.block.x + j * BLOCK_SIZE) // BLOCK_SIZE] != 0:
return True
return False
def freeze(self):
for i in range(len(self.block.shape)):
for j in range(len(self.block.shape[i])):
if self.block.shape[i][j] == 1:
self.grid[(self.block.y + i * BLOCK_SIZE) // BLOCK_SIZE][(self.block.x + j * BLOCK_SIZE) // BLOCK_SIZE] = self.block.color
def clear_lines(self):
lines = []
for i in range(len(self.grid)):
if all(self.grid[i]):
lines.append(i)
for line in lines:
del self.grid[line]
self.grid.insert(0, [0] * (WINDOW_WIDTH // BLOCK_SIZE))
self.score += 1
def draw(self):
self.surface.fill((0, 0, 0))
for i in range(len(self.grid)):
for j in range(len(self.grid[i])):
if self.grid[i][j] != 0:
pygame.draw.rect(self.surface, self.grid[i][j], (j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
self.block.draw(self.surface)
pygame.display.update()
def run(self):
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
self.block.move_left()
if self.collide():
self.block.move_right()
elif event.key == pygame.K_RIGHT:
self.block.move_right()
if self.collide():
self.block.move_left()
elif event.key == pygame.K_DOWN:
self.block.move_down()
if self.collide():
self.block.move_up()
self.freeze()
self.clear_lines()
self.block = Block()
elif event.key == pygame.K_UP:
self.block.rotate()
if self.collide():
self.block.rotate_back()
self.update()
self.draw()
self.clock.tick(5)
pygame.quit()
if __name__ == "__main__":
game = Game()
game.run()
```
相关推荐
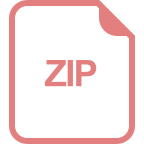
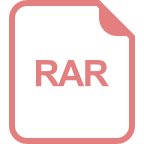















