系统功能基本要求:职工信息,包括职工编号、职工姓名、性别、年龄、职称等;出勤记录信息, 包括上班打卡时间,下班打开时间,缺勤记录等;出差信息,包括出差起始时间、结束时间、统计总共 天数等;请假信息,包括请假开始时间,结束时间,统计请假天数等;加班信息,包括加班开始时间、 结束时间、统计加班总时间。 按照此要求给出JDBC代码
时间: 2023-12-19 21:17:01 浏览: 127
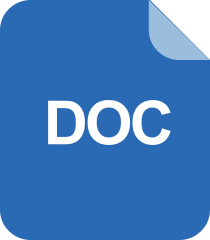
职工信息系统的设计与实现
以下是一个简单的JDBC代码示例,用于连接并操作数据库中的职工信息表:
```java
import java.sql.*;
public class EmployeeJDBC {
private Connection conn = null;
private PreparedStatement pstmt = null;
private ResultSet rs = null;
// 连接数据库
public void connect() throws ClassNotFoundException, SQLException {
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/employees";
String user = "root";
String password = "password";
conn = DriverManager.getConnection(url, user, password);
}
// 插入职工信息
public void insertEmployee(Employee employee) throws SQLException {
String sql = "INSERT INTO employee (employee_id, employee_name, gender, age, title) VALUES (?, ?, ?, ?, ?)";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, employee.getEmployeeId());
pstmt.setString(2, employee.getEmployeeName());
pstmt.setString(3, employee.getGender());
pstmt.setInt(4, employee.getAge());
pstmt.setString(5, employee.getTitle());
pstmt.executeUpdate();
}
// 查询职工信息
public Employee getEmployee(int employeeId) throws SQLException {
String sql = "SELECT * FROM employee WHERE employee_id = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, employeeId);
rs = pstmt.executeQuery();
if (rs.next()) {
Employee employee = new Employee();
employee.setEmployeeId(rs.getInt("employee_id"));
employee.setEmployeeName(rs.getString("employee_name"));
employee.setGender(rs.getString("gender"));
employee.setAge(rs.getInt("age"));
employee.setTitle(rs.getString("title"));
return employee;
} else {
return null;
}
}
// 更新职工信息
public void updateEmployee(Employee employee) throws SQLException {
String sql = "UPDATE employee SET employee_name = ?, gender = ?, age = ?, title = ? WHERE employee_id = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, employee.getEmployeeName());
pstmt.setString(2, employee.getGender());
pstmt.setInt(3, employee.getAge());
pstmt.setString(4, employee.getTitle());
pstmt.setInt(5, employee.getEmployeeId());
pstmt.executeUpdate();
}
// 删除职工信息
public void deleteEmployee(int employeeId) throws SQLException {
String sql = "DELETE FROM employee WHERE employee_id = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, employeeId);
pstmt.executeUpdate();
}
// 关闭连接
public void close() throws SQLException {
if (rs != null) {
rs.close();
}
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
}
}
```
其中,`Employee`类为职工信息类,包括职工编号、职工姓名、性别、年龄、职称等属性和对应的get/set方法。在该示例中,我们使用了预处理语句来执行SQL语句,避免了SQL注入攻击。同时,我们还提供了连接、插入、查询、更新和删除职工信息的方法,以及关闭连接的方法。
阅读全文
相关推荐
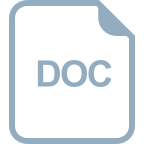
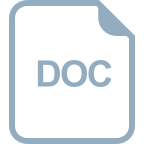
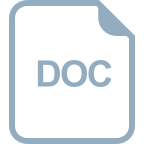
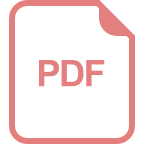
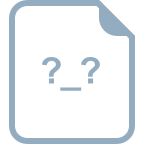
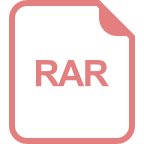
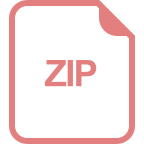
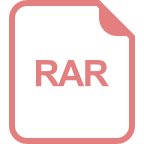
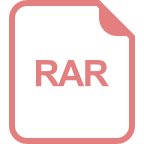
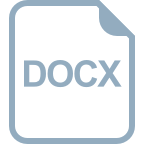
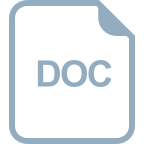
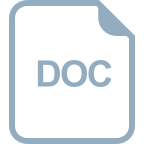
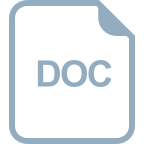
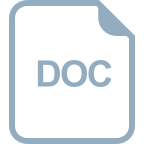
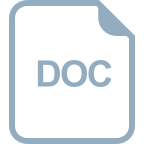
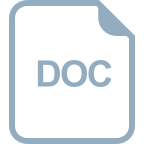
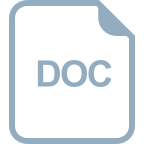
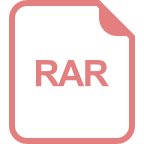
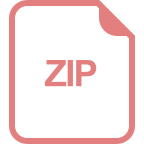