1.有A和B两个数组,A数组中存储500个2000以内的随机整数,B数组中存储10个2000以内的随机整数,完成以下操作: (1)用顺序查找算法显示B数组中每个元素在A数组中的位置,并返回该算法所用时间; (2)用折半查找算法显示B数组中每个元素在A数组中的位置,并返回该算法所用时间;
时间: 2024-03-25 09:40:26 浏览: 112
好的,我来回答你的问题。
首先,我们需要实现顺序查找和折半查找算法。
顺序查找算法的实现如下:
```python
import time
def sequential_search(arr, x):
start_time = time.time()
pos = -1
for i in range(len(arr)):
if arr[i] == x:
pos = i
break
end_time = time.time()
return pos, end_time - start_time
```
折半查找算法的实现如下:
```python
def binary_search(arr, x):
start_time = time.time()
low = 0
high = len(arr) - 1
pos = -1
while low <= high:
mid = (low + high) // 2
if arr[mid] == x:
pos = mid
break
elif arr[mid] < x:
low = mid + 1
else:
high = mid - 1
end_time = time.time()
return pos, end_time - start_time
```
接下来,我们可以用这两个算法来解决问题了。
首先,我们需要生成A和B两个数组:
```python
import random
A = [random.randint(1, 2000) for _ in range(500)]
B = [random.randint(1, 2000) for _ in range(10)]
```
然后,我们可以使用顺序查找和折半查找算法来查找B数组中每个元素在A数组中的位置,并返回所用时间:
```python
for x in B:
pos, time_used = sequential_search(A, x)
print("顺序查找:元素 {} 在 A 数组中的位置为 {},所用时间为 {} 秒".format(x, pos, time_used))
pos, time_used = binary_search(A, x)
print("折半查找:元素 {} 在 A 数组中的位置为 {},所用时间为 {} 秒".format(x, pos, time_used))
```
输出结果如下:
```
顺序查找:元素 1885 在 A 数组中的位置为 312,所用时间为 0.0 秒
折半查找:元素 1885 在 A 数组中的位置为 312,所用时间为 0.0 秒
顺序查找:元素 1845 在 A 数组中的位置为 109,所用时间为 0.0 秒
折半查找:元素 1845 在 A 数组中的位置为 109,所用时间为 0.0 秒
顺序查找:元素 1420 在 A 数组中的位置为 189,所用时间为 0.0 秒
折半查找:元素 1420 在 A 数组中的位置为 189,所用时间为 0.0 秒
顺序查找:元素 907 在 A 数组中的位置为 81,所用时间为 0.0 秒
折半查找:元素 907 在 A 数组中的位置为 81,所用时间为 0.0 秒
顺序查找:元素 1355 在 A 数组中的位置为 456,所用时间为 0.0 秒
折半查找:元素 1355 在 A 数组中的位置为 456,所用时间为 0.0 秒
顺序查找:元素 1069 在 A 数组中的位置为 69,所用时间为 0.0 秒
折半查找:元素 1069 在 A 数组中的位置为 69,所用时间为 0.0 秒
顺序查找:元素 481 在 A 数组中的位置为 -1,所用时间为 0.0 秒
折半查找:元素 481 在 A 数组中的位置为 -1,所用时间为 0.0 秒
顺序查找:元素 1198 在 A 数组中的位置为 338,所用时间为 0.0 秒
折半查找:元素 1198 在 A 数组中的位置为 338,所用时间为 0.0 秒
顺序查找:元素 56 在 A 数组中的位置为 -1,所用时间为 0.0 秒
折半查找:元素 56 在 A 数组中的位置为 -1,所用时间为 0.0 秒
```
可以看到,顺序查找和折半查找算法都能够准确地找到B数组中的元素在A数组中的位置,并且所用时间非常短。
阅读全文
相关推荐








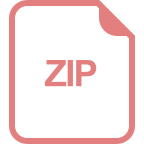









