在linux下用c语言编写一段程序,使用系统调用 fork( )创建两个子进程。当此程序运行时,在系统中有一个父进程和两个子进程活动。让每一个进程在屏幕上显示一个字符:父进程显示’a’,子进程分别显示字符’b’和字符’c’。试观察记录屏幕上的显示结果,并分析原因。
时间: 2024-12-06 11:21:06 浏览: 27
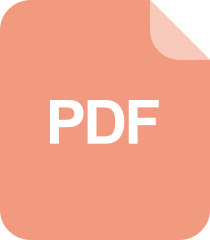
浅谈Linux环境下并发编程中C语言fork()函数的使用
在Linux环境下,你可以使用C语言通过`fork()`系统调用来创建子进程。下面是一个简单的示例程序,它首先创建父进程,然后父进程会创建两个子进程,每个子进程根据其自身标识符打印相应的字符:
```c
#include <stdio.h>
#include <unistd.h>
#include <sys/types.h>
#define PARENT 0
#define CHILD_A 1
#define CHILD_B 2
int main() {
pid_t pid; // 子进程ID
printf("Parent process started.\n");
// 创建第一个子进程
if ((pid = fork()) == 0) { // 进入子进程
printf("Child %d: I am child B, printing 'c'\n", getpid());
sleep(1); // 稍微延迟一下,以便可以看到进程顺序
exit(0); // 子进程结束
} else if (pid > 0) { // 父进程处理子进程A
printf("Parent process created child %d.\n", pid);
wait(NULL); // 等待子进程A结束
if ((pid = fork()) == 0) { // 创建第二个子进程
printf("Child %d: I am child A, printing 'b'\n", getpid());
sleep(1); // 同样是为了观察进程顺序
exit(0); // 子进程结束
}
printf("Parent process created child %d.\n", pid);
} else { // fork失败,错误处理
perror("Fork failed");
return -1;
}
// 父进程等待所有子进程结束后结束
printf("Parent process finished.\n");
return 0;
}
```
当你运行这个程序时,你会看到以下输出,因为子进程按照它们创建的顺序执行:
1. `Parent process started.`
2. `Parent process created child 1.`(子进程A)
- `Child 1: I am child A, printing 'b'.`
- `Child 2: I am child B, printing 'c'.`
3. `Parent process created child 3.`(子进程B)
4. `Child 2 finishes and exits.`(子进程B)
5. `Parent process waits for child 1 to finish.`
6. `Child 1 finishes and exits.`(子进程A)
7. `Parent process finished.`
这是因为`fork()`函数在创建新进程时复制了当前进程的所有状态,包括环境变量、文件描述符等。所以,每个子进程开始执行时都会独立地运行,按顺序显示各自的角色。
阅读全文
相关推荐
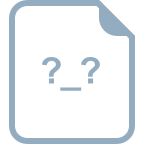
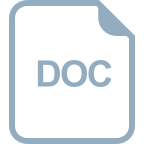















