class Demo{ public static void main(String []s){ int a = 5, b = 0; try{a = a/b;} catch(Exception e){System.out.print(“异常”)} catch(AirthmeticException e){ System.out.print(“算术异常”); } Catch(IndexOutOfBoundsException e){ System.out.print(“越界异常”); } }} 则程序运行结果为( ) A 显示:异常 B 显示:算术异常 C 显示:越界异常 D 编译错误
时间: 2024-03-01 08:53:21 浏览: 5
程序存在编译错误,因为 `catch` 块应该按照从小到大的顺序排列(即从子类到父类),因为子类异常会被父类异常所捕获。正确的代码如下:
```java
class Demo{
public static void main(String []s){
int a = 5, b = 0;
try {
a = a/b;
} catch (ArithmeticException e) {
System.out.print("算术异常");
} catch (IndexOutOfBoundsException e) {
System.out.print("越界异常");
} catch (Exception e) {
System.out.print("异常");
}
}
}
```
运行结果为 B:显示“算术异常”,因为程序在执行 `a = a/b` 时会抛出除数为零的算术异常,被第一个 `catch` 块所捕获。
相关问题
public class Demo { public static void main(String[] args) { int num = 10; System.out.println(test (num)) ; 7 public static int test (int b) { try { b += 10; return b; } catch (RuntimeException e) { } catch (Exception e2) { ] finally { b += 10; return b; } }
这段代码是有问题的,编译器会提示错误信息。
首先,在test方法中,try和catch语句块都没有返回语句,因此编译器会提示缺少返回语句的错误。另外,在finally语句块中也有一个返回语句,这样会导致try或catch语句块中的返回语句被忽略,因为finally语句块中的返回语句会覆盖try或catch语句块中的返回语句。
因此,如果想要修复这段代码,需要在finally语句块中移除返回语句,或者在try或catch语句块中添加一个返回语句。例如,可以将finally语句块中的return b;改为b += 10;。
修复后的代码如下:
public class Demo {
public static void main(String[] args) {
int num = 10;
System.out.println(test(num));
}
public static int test(int b) {
try {
b += 10;
return b;
} catch (RuntimeException e) {
} catch (Exception e2) {
} finally {
b += 10;
}
return b;
}
}
这样,输出结果为30。
public class Demo { public static void main(String[] args) { int num = 10; System.out.println(test(num)); } public static int test(int b) { try { b += 10; return b; } catch (RuntimeException e) { } catch (Exception e2) { } finally { b += 10; } return b; } }
这段代码的输出结果是20。
在test方法中,try语句块中的b += 10;语句会执行,将b的值变为20。然后,return语句将b的值20返回给调用者。
在finally语句块中,b += 10;语句也会执行,将b的值再次加10,变为30。但是,由于return语句已经将b的值返回给调用者,因此finally语句块中的b += 10;语句并不会影响输出结果。
因此,输出结果为20。
相关推荐
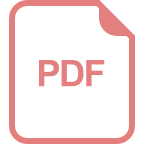
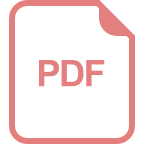
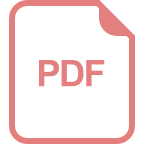












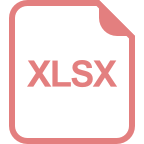