基于Androidstudio的蓝牙通信服务端代码
时间: 2024-05-05 11:23:02 浏览: 155
以下是一个基于 Android Studio 的蓝牙通信服务端代码的示例:
```java
public class BluetoothServer extends Thread {
private final BluetoothServerSocket mmServerSocket;
private BluetoothAdapter mBluetoothAdapter;
private Context mContext;
private Handler mHandler;
private BluetoothSocket mBluetoothSocket;
public BluetoothServer(Context context, Handler handler) {
mContext = context;
mHandler = handler;
mBluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
BluetoothServerSocket tmp = null;
try {
// MY_UUID is the app's UUID string, also used by the client code.
tmp = mBluetoothAdapter.listenUsingRfcommWithServiceRecord("BluetoothServer", MY_UUID);
} catch (IOException e) {
Log.e(TAG, "Socket's listen() method failed", e);
}
mmServerSocket = tmp;
}
public void run() {
mBluetoothSocket = null;
// Keep listening until exception occurs or a socket is returned.
while (true) {
try {
mBluetoothSocket = mmServerSocket.accept();
} catch (IOException e) {
Log.e(TAG, "Socket's accept() method failed", e);
break;
}
if (mBluetoothSocket != null) {
// A connection was accepted. Perform work associated with
// the connection in a separate thread.
manageMyConnectedSocket(mBluetoothSocket);
try {
mmServerSocket.close();
} catch (IOException e) {
Log.e(TAG, "Could not close the connect socket", e);
}
break;
}
}
}
// Closes the connect socket and causes the thread to finish.
public void cancel() {
try {
mBluetoothSocket.close();
} catch (IOException e) {
Log.e(TAG, "Could not close the connect socket", e);
}
}
private void manageMyConnectedSocket(BluetoothSocket socket) {
// Do work to manage the connection (in a separate thread)
// For example:
// InputStream in = socket.getInputStream();
// OutputStream out = socket.getOutputStream();
}
}
```
在这个示例中,我们创建了一个名为 `BluetoothServer` 的线程,在 `run()` 方法中,我们使用 `listenUsingRfcommWithServiceRecord()` 方法来创建一个蓝牙服务器端的 socket,然后调用 `accept()` 方法来监听客户端的连接请求。如果有客户端连接成功,`accept()` 方法会返回一个已连接的蓝牙 socket,我们可以在 `manageMyConnectedSocket()` 方法中处理连接。
要使用这个服务端代码,您需要在您的应用程序中创建一个 `BluetoothServer` 的实例,并将其启动:
```java
BluetoothServer server = new BluetoothServer(this, mHandler);
server.start();
```
其中,`mHandler` 是用于在主线程中处理蓝牙连接事件的 `Handler` 对象。
请注意,这只是一个示例代码,您需要根据您的实际需求进行修改和扩展。
阅读全文
相关推荐
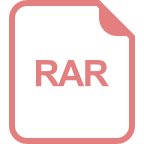
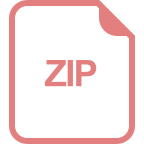
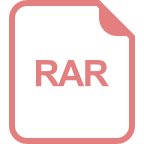
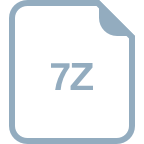
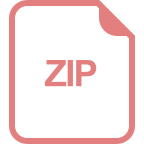
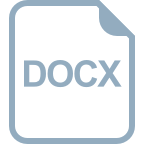
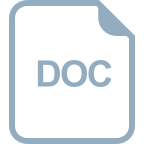
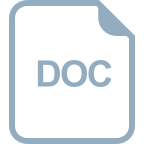
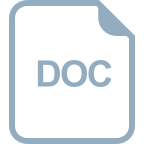
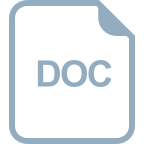
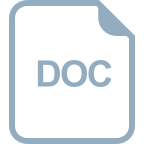
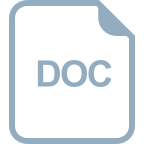
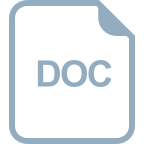
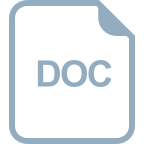
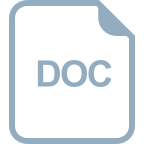
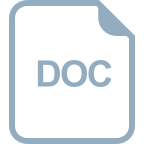
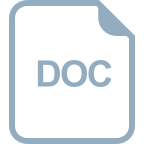