python程序暂停和继续
时间: 2023-06-26 07:10:35 浏览: 246
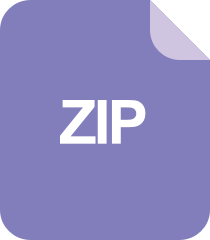
vscode_c_and_python_debug.zip
在Python中,可以使用`time`模块的`sleep()`函数来暂停程序执行。`sleep()`函数的参数为暂停的时间,单位为秒。
例如,以下代码将暂停程序执行3秒钟:
```python
import time
print("开始执行")
time.sleep(3)
print("暂停3秒钟后继续执行")
```
如果需要让程序在某个条件下暂停执行,可以使用`while`循环结合`sleep()`函数来实现。例如,以下代码将每隔1秒钟打印一次当前时间,直到程序按下`Ctrl+C`结束:
```python
import time
try:
while True:
print(time.strftime("%Y-%m-%d %H:%M:%S", time.localtime()))
time.sleep(1)
except KeyboardInterrupt:
print("程序已结束")
```
如果需要在程序运行时动态地控制程序的暂停和继续,可以使用`threading`模块中的`Event`对象。`Event`对象可以用来协调不同线程之间的操作。
下面是一个使用`Event`对象来控制程序暂停和继续的例子:
```python
import threading
import time
# 创建一个Event对象
pause_event = threading.Event()
def pause():
# 暂停程序执行
pause_event.clear()
print("程序已暂停")
def resume():
# 继续程序执行
pause_event.set()
print("程序已继续")
def do_something():
while True:
# 检测Event对象是否被设置
if pause_event.is_set():
print(time.strftime("%Y-%m-%d %H:%M:%S", time.localtime()))
# 暂停一段时间
time.sleep(1)
# 启动一个线程来执行任务
thread = threading.Thread(target=do_something)
thread.start()
# 在主线程中控制程序的暂停和继续
while True:
command = input("请输入指令(pause/resume):")
if command == "pause":
pause()
elif command == "resume":
resume()
else:
print("无效指令")
```
阅读全文
相关推荐
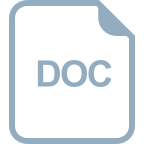
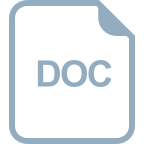


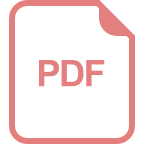












