Django实现文章三级分类,并显示指定分类
时间: 2024-01-06 21:04:37 浏览: 52
可以通过 Django 的 models 实现文章的三级分类,具体步骤如下:
1. 定义三个 model 类,分别表示三个级别的分类,每个类中包含字段:名称、父级分类、是否为叶子节点等。
2. 在文章的 model 类中,添加一个外键字段,关联到三级分类的叶子节点。
3. 在视图中,通过查询参数获取指定的分类,并传递给模板。
4. 在模板中,通过分类的父子关系递归显示分类,并在页面中展示相关文章。
以下是示例代码:
```python
# models.py
class Category(models.Model):
name = models.CharField(max_length=50)
parent = models.ForeignKey('self', null=True, blank=True, related_name='children', on_delete=models.CASCADE)
is_leaf_node = models.BooleanField(default=False)
# Article model
class Article(models.Model):
title = models.CharField(max_length=100)
content = models.TextField()
category = models.ForeignKey(Category, on_delete=models.CASCADE)
# views.py
def article_list(request):
category_id = request.GET.get('category')
if category_id:
category = Category.objects.get(id=category_id)
articles = Article.objects.filter(category__in=category.get_descendants(include_self=True))
else:
articles = Article.objects.all()
return render(request, 'article_list.html', {'articles': articles, 'category': category})
# article_list.html
{% if category %}
<h2>{{ category }}</h2>
{% endif %}
<ul>
{% for child in category.get_children %}
<li>
{{ child }}
<ul>
{% for subchild in child.get_children %}
<li>{{ subchild }}</li>
{% endfor %}
</ul>
</li>
{% endfor %}
</ul>
{% for article in articles %}
<h3>{{ article.title }}</h3>
<p>{{ article.content }}</p>
{% endfor %}
```
注意,以上代码仅为示例,实际情况可能需要根据具体需求进行调整。
相关推荐
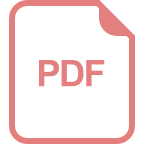














