哲学家进餐问题的c++代码
时间: 2023-07-16 16:13:10 浏览: 78
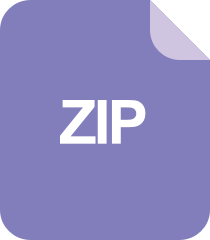
哲学家进餐问题的代码
哲学家进餐问题是一个经典的同步问题,其关键在于如何实现线程之间的同步与互斥。下面是一个简单的 C++ 代码实现,使用了互斥锁和条件变量来保证线程之间的同步。
```c++
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
using namespace std;
const int N = 5; // 哲学家数量
mutex forks[N]; // 叉子互斥锁
condition_variable cv[N]; // 条件变量,用于线程同步
void philosopher(int i) {
while (true) {
// 模拟思考
this_thread::sleep_for(chrono::milliseconds(rand() % 1000));
cout << "Philosopher " << i << " is hungry." << endl;
// 拿起左手的叉子
forks[i].lock();
cout << "Philosopher " << i << " picks up left fork." << endl;
// 尝试拿起右手的叉子
unique_lock<mutex> lock(forks[(i + 1) % N], defer_lock);
while (!lock.try_lock()) {
cv[i].wait(lock);
}
cout << "Philosopher " << i << " picks up right fork." << endl;
// 进餐
cout << "Philosopher " << i << " starts eating." << endl;
this_thread::sleep_for(chrono::milliseconds(rand() % 1000));
cout << "Philosopher " << i << " finishes eating." << endl;
// 放下右手的叉子
lock.unlock();
cv[(i + 1) % N].notify_one();
cout << "Philosopher " << i << " puts down right fork." << endl;
// 放下左手的叉子
forks[i].unlock();
cout << "Philosopher " << i << " puts down left fork." << endl;
}
}
int main() {
thread philosophers[N];
for (int i = 0; i < N; i++) {
philosophers[i] = thread(philosopher, i);
}
for (int i = 0; i < N; i++) {
philosophers[i].join();
}
return 0;
}
```
这个代码中,每个哲学家都是一个线程,通过互斥锁和条件变量来确保只有拿到两个叉子的哲学家才可以进餐,避免了死锁的情况。同时,代码中使用了随机数来模拟哲学家思考和进餐的时间,以增加随机性和趣味性。
阅读全文
相关推荐

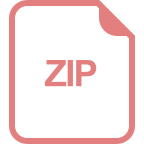





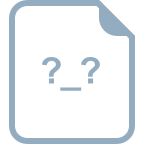
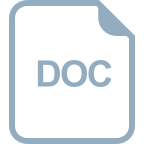
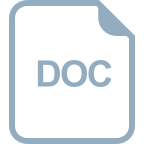
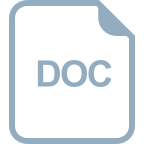

