至多允许4名哲学家同时进餐的C++代码
时间: 2023-07-16 10:11:42 浏览: 48
这是一个经典的哲学家就餐问题,可以使用信号量来实现。
```c++
#include <iostream>
#include <thread>
#include <mutex>
#include <chrono>
#include <semaphore.h>
using namespace std;
const int NUM_PHILOSOPHERS = 5;
sem_t forks[NUM_PHILOSOPHERS];
mutex printMutex;
void philosopher(int id) {
while (true) {
printMutex.lock();
cout << "Philosopher " << id << " is thinking." << endl;
printMutex.unlock();
// Wait for left fork
sem_wait(&forks[id]);
printMutex.lock();
cout << "Philosopher " << id << " picked up left fork." << endl;
printMutex.unlock();
// Wait for right fork
sem_wait(&forks[(id + 1) % NUM_PHILOSOPHERS]);
printMutex.lock();
cout << "Philosopher " << id << " picked up right fork." << endl;
printMutex.unlock();
// Eating
printMutex.lock();
cout << "Philosopher " << id << " is eating." << endl;
printMutex.unlock();
this_thread::sleep_for(chrono::seconds(2));
// Release forks
sem_post(&forks[id]);
printMutex.lock();
cout << "Philosopher " << id << " put down left fork." << endl;
printMutex.unlock();
sem_post(&forks[(id + 1) % NUM_PHILOSOPHERS]);
printMutex.lock();
cout << "Philosopher " << id << " put down right fork." << endl;
printMutex.unlock();
}
}
int main() {
// Initialize forks
for (int i = 0; i < NUM_PHILOSOPHERS; i++) {
sem_init(&forks[i], 0, 1);
}
// Create philosopher threads
thread philosophers[NUM_PHILOSOPHERS];
for (int i = 0; i < NUM_PHILOSOPHERS; i++) {
philosophers[i] = thread(philosopher, i);
}
// Wait for philosopher threads to finish
for (int i = 0; i < NUM_PHILOSOPHERS; i++) {
philosophers[i].join();
}
// Destroy forks
for (int i = 0; i < NUM_PHILOSOPHERS; i++) {
sem_destroy(&forks[i]);
}
return 0;
}
```
在这个代码中,我们使用了一个信号量数组 `forks` 来表示每个叉子的可用性。每个哲学家线程在循环中执行以下步骤:
1. 思考一会儿(两秒钟)。
2. 等待左边的叉子。
3. 等待右边的叉子。
4. 吃饭(两秒钟)。
5. 放下左边的叉子。
6. 放下右边的叉子。
`sem_wait` 和 `sem_post` 分别用于等待和释放信号量。在这个问题中,每个哲学家需要同时拿到左边和右边的叉子才能开始吃饭。如果叉子不可用,`sem_wait` 会阻塞该线程,直到叉子可用。
由于哲学家可能会陷入死锁,因此需要使用某种机制来避免死锁。这里我们使用了一个简单的规则:每个哲学家先拿起左边的叉子,然后再去尝试拿右边的叉子。这样可以避免所有哲学家同时拿起左边的叉子,导致右边的叉子都被占用,从而导致死锁。
相关推荐
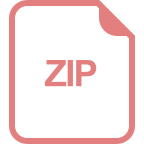














