### Problem 2: Repeated Implement `repeated`, which takes in an iterator `t` and returns the first value in `t` that appears `k` times in a row. > **Note:** You can assume that the iterator `t` will have a value that appears at least `k` times in a row. If you are receiving a `StopIteration`, your `repeated` function is likely not identifying the correct value. Your implementation should iterate through the items in a way such that if the same iterator is passed into `repeated` twice, it should continue in the second call at the point it left off in the first. An example of this behavior is in the doctests. ```python def repeated(t, k): """Return the first value in iterator T that appears K times in a row. Iterate through the items such that if the same iterator is passed into the function twice, it continues in the second call at the point it left off in the first. >>> s = iter([10, 9, 10, 9, 9, 10, 8, 8, 8, 7]) >>> repeated(s, 1) 10 >>> repeated(s, 2) 9 >>> s2 = iter([10, 9, 10, 9, 9, 10, 8, 8, 8, 7]) >>> repeated(s2, 3) 8 >>> s = iter([3, 2, 2, 2, 2, 2, 2, 4, 4, 5, 5, 5]) >>> repeated(s, 3) 2 >>> repeated(s, 3) 2 >>> repeated(s, 3) 5 >>> s2 = iter([4, 1, 6, 6, 7, 7, 8, 8, 2, 2, 2, 5]) >>> repeated(s2, 3) 2 """ assert k >= 1 "*** YOUR CODE HERE ***" ```
时间: 2023-07-15 19:09:55 浏览: 111
一个简单的思路是,利用一个计数器count记录当前值连续出现的次数,以及一个变量previous记录前一个值,如果当前值与前一个值相同,则count+1,否则count重置为1。当count等于k时,说明找到了连续的k个相同的值,返回该值即可。需要注意的是,如果在函数执行过程中,迭代器t已经到达了末尾,则需要将其重置为起始状态,以便后续调用函数时能够继续使用。代码如下:
相关问题
Implement repeated, which takes in an iterator t and returns the first value in t that appears k times in a row.
Sure, here's a possible implementation of the `repeated` function in Python:
```python
def repeated(t, k):
last = None
count = 0
for item in t:
if item == last:
count += 1
else:
last = item
count = 1
if count == k:
return item
return None
```
The `repeated` function takes two arguments: `t` is the iterator we want to search for repeated values, and `k` is the number of times a value must appear in a row to be considered repeated. The function initializes a `last` variable to `None` to keep track of the last value seen, and a `count` variable to 0 to keep track of the number of times the last value has been seen in a row. The function iterates over the items in `t` and updates the `last` and `count` variables accordingly. If the `count` variable reaches `k`, the function returns the current item (i.e., we have found a repeated value). If we reach the end of the iterator without finding a repeated value, the function returns `None`.
For example, we can use the function like this:
```python
it = iter([1, 2, 3, 3, 3, 4, 4, 5, 5, 5, 5])
print(repeated(it, 3)) # Output: 3
```
In this example, we create an iterator `it` over a list with repeated values. We then call `repeated(it, 3)` to search for a value that appears 3 times in a row, and it returns 3.
用c语言解决下列问题:程序:检查数中重复出现的数字。 用户输入数后,程序显示信息Repeated digit或No Repeated digit: Enter a number: 28212 Repeated digit 数28212有一个重复的数字(2),而数9357则没有。 **输入格式要求:"%ld" 提示信息:"Enter a number :" **输出格式要求:"Repeated digit\n\n" "No Repeated digit\n\n" 程序运行示例1: Enter a number :67 No Repeated digit 程序运行示例2: Enter a number :2556 Repeated digit
下面是C语言的程序代码:
```c
#include <stdio.h>
int main() {
long num;
int digit_seen[10] = {0};
int has_repeated_digit = 0;
printf("Enter a number: ");
scanf("%ld", &num);
while(num > 0) {
int digit = num % 10;
if(digit_seen[digit]) {
has_repeated_digit = 1;
break;
} else {
digit_seen[digit] = 1;
}
num /= 10;
}
if(has_repeated_digit) {
printf("Repeated digit\n\n");
} else {
printf("No Repeated digit\n\n");
}
return 0;
}
```
程序运行时,先读取用户输入的数值,然后使用一个整型数组digit_seen来记录每个数字是否出现过。遍历输入的数值每一位的数字,如果已经出现过,则将has_repeated_digit标志设置为1,并跳出循环。最后根据has_repeated_digit的值输出相应的信息。
阅读全文
相关推荐
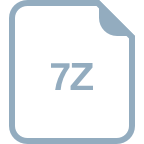
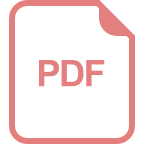
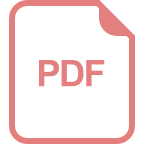












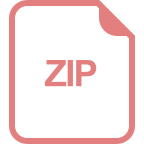