java实现红黑树插入删除代码
时间: 2023-02-08 17:03:33 浏览: 78
红黑树是一种自平衡二叉查找树。它的插入和删除操作都可能导致树的不平衡,需要进行旋转和重新着色操作来维护树的平衡。
下面是一个 Java 程序,它实现了红黑树的插入和删除操作:
```java
public class RedBlackTree<T extends Comparable<T>> {
private static final boolean RED = true;
private static final boolean BLACK = false;
private Node<T> root;
private class Node<T extends Comparable<T>> {
T key;
Node<T> left, right;
boolean color;
Node(T key, boolean color) {
this.key = key;
this.color = color;
}
}
private boolean isRed(Node<T> x) {
if (x == null) return false;
return x.color == RED;
}
private Node<T> rotateLeft(Node<T> h) {
Node<T> x = h.right;
h.right = x.left;
x.left = h;
x.color = h.color;
h.color = RED;
return x;
}
private Node<T> rotateRight(Node<T> h) {
Node<T> x = h.left;
h.left = x.right;
x.right = h;
x.color = h.color;
h.color = RED;
return x;
}
private void flipColors(Node<T> h) {
h.color = !h.color;
h.left.color = !h.left.color;
h.right.color = !h.right.color;
}
public void insert(T key) {
root = insert(root, key);
root.color = BLACK;
}
private Node<T> insert(Node<T> h, T key) {
if (h == null) return new Node<>(key, RED);
int cmp = key.compareTo(h.key);
if (cmp < 0) h.left = insert(h.left, key);
else if (cmp > 0) h.right = insert(h.right, key);
else h.key = key;
if (isRed(h.right) && !isRed(h.left)) h = rotateLeft(h);
if (isRed(h.left) && isRed(h.left.left)) h = rotateRight(h);
if (isRed(h.left) && isRed(h.right)) flipColors(h);
return h;
}
public void delete
相关推荐
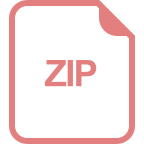
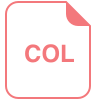
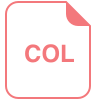
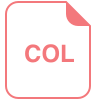
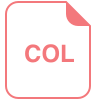
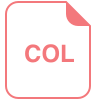









