如何用java代码读取在MySQL导出的CSV文件并在VUE上面用高德地图表示出来
时间: 2024-03-23 09:43:39 浏览: 164
要用Java代码读取MySQL导出的CSV文件并在Vue上使用高德地图表示它们,您需要完成以下步骤:
1. 使用Java代码读取CSV文件并将其转换为Java对象。
2. 使用Java对象中的地理位置信息调用高德地图Web服务API,将其转换为高德地图中的经纬度坐标。
3. 将转换后的数据作为JSON格式的数据传递给Vue组件,并在Vue组件中使用高德地图API将它们在地图上表示。
下面是一个简单的Java代码示例,可以将包含地理位置信息的CSV文件转换为高德地图中的经纬度坐标,并将它们作为JSON格式的数据传递给Vue组件:
```java
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import com.amap.api.maps.model.LatLng;
public class LocationLoader {
private static final String CSV_FILE = "locations.csv";
private static final String API_KEY = "your_api_key";
public static void main(String[] args) {
// 读取CSV文件
List<Location> locations = readCsvFile(CSV_FILE);
// 将地理位置信息转换为经纬度坐标
for (Location location : locations) {
LatLng latLng = geocodeLocation(location.getAddress());
location.setLatitude(latLng.latitude);
location.setLongitude(latLng.longitude);
}
// 将转换后的数据作为JSON格式的数据传递给Vue组件
JSONArray jsonArray = new JSONArray();
for (Location location : locations) {
JSONObject jsonObject = new JSONObject();
jsonObject.put("name", location.getName());
jsonObject.put("address", location.getAddress());
jsonObject.put("latitude", location.getLatitude());
jsonObject.put("longitude", location.getLongitude());
jsonArray.add(jsonObject);
}
System.out.println(jsonArray.toJSONString());
}
private static List<Location> readCsvFile(String fileName) {
List<Location> locations = new ArrayList<>();
try (BufferedReader br = new BufferedReader(new FileReader(fileName))) {
String line;
while ((line = br.readLine()) != null) {
String[] values = line.split(",");
locations.add(new Location(values[0], values[1]));
}
} catch (IOException e) {
e.printStackTrace();
}
return locations;
}
private static LatLng geocodeLocation(String address) {
AMap amap = new AMap(API_KEY);
JSONObject json = amap.geocode(address);
return new LatLng(json.getJSONObject("location").getDouble("lat"), json.getJSONObject("location").getDouble("lng"));
}
}
class Location {
private String name;
private String address;
private double latitude;
private double longitude;
public Location(String name, String address) {
this.name = name;
this.address = address;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public double getLatitude() {
return latitude;
}
public void setLatitude(double latitude) {
this.latitude = latitude;
}
public double getLongitude() {
return longitude;
}
public void setLongitude(double longitude) {
this.longitude = longitude;
}
}
```
在上面的代码中,我们使用了高德地图的Java SDK(amap)来将地理位置信息转换为经纬度坐标,需要先在高德地图开发者中心申请API Key,并将其替换为代码中的“your_api_key”部分。
完成了上述步骤后,您可以将转换后的JSON格式的数据传递给Vue组件,例如:
```javascript
<template>
<div id="map-container"></div>
</template>
<script>
import AMapLoader from '@amap/amap-jsapi-loader';
export default {
name: 'MapComponent',
async mounted() {
// 加载高德地图API
await AMapLoader.load({
key: 'your_api_key',
version: '2.0',
plugins: ['AMap.Marker'],
});
// 加载地理位置信息
const response = await fetch('locations.json');
const locations = await response.json();
// 在地图上添加标记
const map = new AMap.Map('map-container', {
zoom: 10,
});
locations.forEach((location) => {
const marker = new AMap.Marker({
position: [location.longitude, location.latitude],
title: location.name,
});
marker.setMap(map);
});
},
};
</script>
```
在上面的代码中,我们使用了@amap/amap-jsapi-loader库来异步加载高德地图API,并在Vue组件中使用高德地图API将地理位置信息在地图上表示。需要先在高德地图开发者中心申请API Key,并将其替换为代码中的“your_api_key”部分。
请注意,在使用高德地图的API时,需要遵守高德地图的相关使用条款和隐私政策。
阅读全文
相关推荐
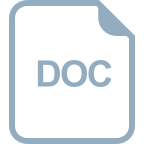
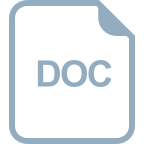
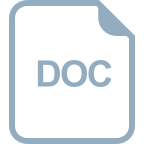
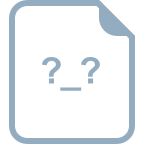
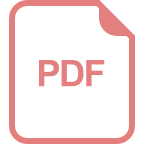
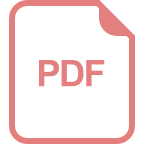
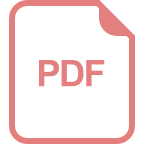
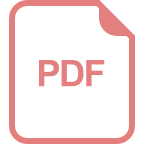
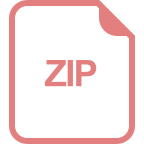
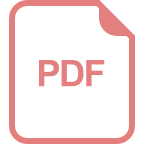
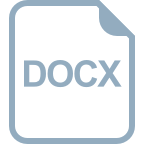
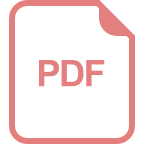
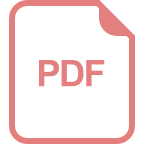
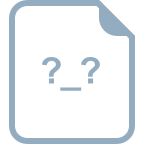
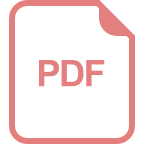
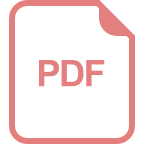
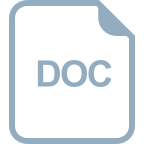
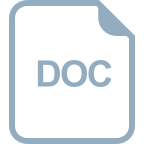
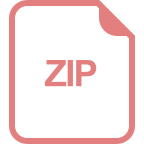