我的数据库表结构式这样的 t_customer: id-username-password 1-admin-$2a$10$YPsBxlg8QeNtLcmEDiH4qO5WAwJLmar/4BKuzmfu3MH/GYTCYM2ae 2-user-$2a$10$0KcJzxj8JYd0EVau1RLud.YbHeINx/xu3fEp.OYeXEPoU6DlqpJOW t_authority id-authority 1-ADMIN 2-USER 根据此数据库写一个自定义认证SecurityConfig
时间: 2023-08-12 11:05:03 浏览: 190
好的,以下是一个示例的自定义认证SecurityConfig:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private CustomUserDetailsService customUserDetailsService;
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasAuthority("ADMIN")
.antMatchers("/user/**").hasAnyAuthority("ADMIN", "USER")
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(customUserDetailsService)
.passwordEncoder(passwordEncoder());
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
```
其中,`CustomUserDetailsService` 是自定义的用户信息服务类,用于查询用户信息和权限信息。我们可以根据 `t_customer` 和 `t_authority` 表来实现这个类,下面是一个示例:
```java
@Service
public class CustomUserDetailsService implements UserDetailsService {
@Autowired
private CustomerRepository customerRepository;
@Autowired
private AuthorityRepository authorityRepository;
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
Customer customer = customerRepository.findByUsername(username);
if (customer == null) {
throw new UsernameNotFoundException("User not found");
}
List<Authority> authorities = authorityRepository.findByCustomerId(customer.getId());
List<GrantedAuthority> grantedAuthorities = new ArrayList<>();
for (Authority authority : authorities) {
grantedAuthorities.add(new SimpleGrantedAuthority(authority.getAuthority()));
}
return new User(customer.getUsername(), customer.getPassword(), grantedAuthorities);
}
}
```
在这个示例中,我们使用了 `CustomerRepository` 和 `AuthorityRepository` 来实现对 `t_customer` 和 `t_authority` 表的查询操作。根据用户名查询到对应的用户信息后,我们再根据用户 ID 查询该用户的权限信息,并将权限信息转换为 Spring Security 的 `GrantedAuthority` 对象。最后,我们将查询到的用户信息和权限信息封装为一个 `UserDetails` 对象并返回。
最后,我们需要实现 `CustomerRepository` 和 `AuthorityRepository` 接口来实现对 `t_customer` 和 `t_authority` 表的查询操作。这里我们可以使用 Spring Data JPA 来简化操作,以下是一个示例:
```java
public interface CustomerRepository extends JpaRepository<Customer, Long> {
Customer findByUsername(String username);
}
public interface AuthorityRepository extends JpaRepository<Authority, Long> {
List<Authority> findByCustomerId(Long customerId);
}
```
需要注意的是,`Customer` 和 `Authority` 分别对应 `t_customer` 和 `t_authority` 表的实体类。在实体类中,我们需要使用 `@Table`、`@Id`、`@Column` 等注解来指定表名、主键和字段名。具体实现可以参考以下代码:
```java
@Entity
@Table(name = "t_customer")
public class Customer {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "username")
private String username;
@Column(name = "password")
private String password;
// 省略 getter 和 setter
}
@Entity
@Table(name = "t_authority")
public class Authority {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "authority")
private String authority;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "customer_id")
private Customer customer;
// 省略 getter 和 setter
}
```
在 `Authority` 实体类中,我们使用了 `@ManyToOne` 和 `@JoinColumn` 注解来指定与 `Customer` 表的关联关系。这里使用了懒加载策略(`FetchType.LAZY`),即在查询 `Authority` 表时不会立即查询 `Customer` 表,只有在需要时才会查询。
阅读全文
相关推荐





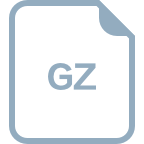











