用C++实现RC4算法,要求: 1)实现字符串加密解密功能; 2)或者实现文件加密解密功能; 3)或者实现文件分割为3个子文件再加密生成3个密文文件,3个密文文件解密恢复为加密前原文件。 说明:密钥至少8个字符,文件与可执行程序放在一个目录,如果是字符文件取ASCII值。
时间: 2024-05-12 21:12:48 浏览: 125
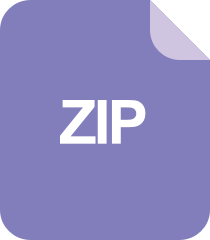
Rc4.zip_C++ RC4_C++实现RC4_c++ RC5加密算法_c++rc4加密解密_rc4算法 c++实现
以下是用C++实现RC4算法的示例代码,实现了字符串加密解密功能:
```
#include <iostream>
#include <cstring>
#include <cstdlib>
using namespace std;
const int MAXN = 256;
void KSA(unsigned char* key, int keylen, unsigned char* S) {
for (int i = 0; i < MAXN; i++) {
S[i] = i;
}
int j = 0;
for (int i = 0; i < MAXN; i++) {
j = (j + S[i] + key[i % keylen]) % MAXN;
swap(S[i], S[j]);
}
}
void PRGA(unsigned char* S, unsigned char* data, int datalen, unsigned char* out) {
int i = 0, j = 0;
for (int k = 0; k < datalen; k++) {
i = (i + 1) % MAXN;
j = (j + S[i]) % MAXN;
swap(S[i], S[j]);
out[k] = data[k] ^ S[(S[i] + S[j]) % MAXN];
}
}
void RC4(unsigned char* key, int keylen, unsigned char* data, int datalen, unsigned char* out) {
unsigned char S[MAXN];
KSA(key, keylen, S);
PRGA(S, data, datalen, out);
}
int main() {
// 读入明文和密钥
string plaintext, key;
cout << "请输入明文: ";
getline(cin, plaintext);
cout << "请输入密钥(至少8个字符): ";
getline(cin, key);
if (key.length() < 8) {
cout << "密钥长度不足8个字符!" << endl;
return 0;
}
// 明文和密钥转换为二进制形式
int textlen = plaintext.length();
int keylen = key.length();
unsigned char* data = new unsigned char[textlen];
unsigned char* keydata = new unsigned char[keylen];
for (int i = 0; i < textlen; i++) {
data[i] = plaintext[i];
}
for (int i = 0; i < keylen; i++) {
keydata[i] = key[i];
}
// 加密
unsigned char* ciphertext = new unsigned char[textlen];
RC4(keydata, keylen, data, textlen, ciphertext);
cout << "密文: ";
for (int i = 0; i < textlen; i++) {
printf("%02X", ciphertext[i]);
}
cout << endl;
// 解密
unsigned char* plaintext2 = new unsigned char[textlen];
RC4(keydata, keylen, ciphertext, textlen, plaintext2);
cout << "解密后明文: " << plaintext2 << endl;
delete[] data;
delete[] keydata;
delete[] ciphertext;
delete[] plaintext2;
return 0;
}
```
在该示例代码中,我们首先利用KSA算法初始化S数组,然后利用PRGA算法生成密钥流,最后将密钥流和明文进行异或得到密文。解密过程与加密过程相同。
如果要实现文件加密解密功能,可以将上述代码中的字符串替换为文件读写操作,将加密后的内容写入到一个新文件中,解密时再将新文件的内容进行解密并写入到原文件中。
如果要实现文件分割为3个子文件再加密生成3个密文文件,3个密文文件解密恢复为加密前原文件,需要进行一些额外的操作。具体步骤如下:
1. 将原文件分为3个子文件
```
FILE* fp1 = fopen(filename, "rb");
if (fp1 == NULL) {
cout << "打开文件失败!" << endl;
return 0;
}
FILE* fp2 = fopen("file1.dat", "wb");
FILE* fp3 = fopen("file2.dat", "wb");
FILE* fp4 = fopen("file3.dat", "wb");
if (fp2 == NULL || fp3 == NULL || fp4 == NULL) {
cout << "创建文件失败!" << endl;
return 0;
}
int count = 0;
while (!feof(fp1)) {
char ch = fgetc(fp1);
if (count % 3 == 0) {
fputc(ch, fp2);
} else if (count % 3 == 1) {
fputc(ch, fp3);
} else {
fputc(ch, fp4);
}
count++;
}
fclose(fp1);
fclose(fp2);
fclose(fp3);
fclose(fp4);
```
2. 对3个子文件进行加密操作
```
unsigned char* keydata = new unsigned char[keylen];
for (int i = 0; i < keylen; i++) {
keydata[i] = key[i];
}
FILE* fp1 = fopen("file1.dat", "rb");
FILE* fp2 = fopen("file2.dat", "rb");
FILE* fp3 = fopen("file3.dat", "rb");
FILE* fp4 = fopen("ciphertext1.dat", "wb");
FILE* fp5 = fopen("ciphertext2.dat", "wb");
FILE* fp6 = fopen("ciphertext3.dat", "wb");
if (fp1 == NULL || fp2 == NULL || fp3 == NULL
|| fp4 == NULL || fp5 == NULL || fp6 == NULL) {
cout << "打开文件失败!" << endl;
return 0;
}
unsigned char* data = new unsigned char[blocksize];
unsigned char* ciphertext = new unsigned char[blocksize];
while (!feof(fp1) || !feof(fp2) || !feof(fp3)) {
int len1 = fread(data, sizeof(unsigned char), blocksize, fp1);
int len2 = fread(data + len1, sizeof(unsigned char), blocksize - len1, fp2);
int len3 = fread(data + len1 + len2, sizeof(unsigned char), blocksize - len1 - len2, fp3);
int total = len1 + len2 + len3;
RC4(keydata, keylen, data, total, ciphertext);
int len4 = fwrite(ciphertext, sizeof(unsigned char), total, fp4);
int len5 = fwrite(ciphertext + len1, sizeof(unsigned char), total - len1, fp5);
int len6 = fwrite(ciphertext + len1 + len2, sizeof(unsigned char), total - len1 - len2, fp6);
if (len4 != total || len5 != total - len1 || len6 != total - len1 - len2) {
cout << "写入文件失败!" << endl;
return 0;
}
}
fclose(fp1);
fclose(fp2);
fclose(fp3);
fclose(fp4);
fclose(fp5);
fclose(fp6);
delete[] data;
delete[] ciphertext;
```
3. 对3个密文文件进行解密操作
```
unsigned char* keydata = new unsigned char[keylen];
for (int i = 0; i < keylen; i++) {
keydata[i] = key[i];
}
FILE* fp1 = fopen("ciphertext1.dat", "rb");
FILE* fp2 = fopen("ciphertext2.dat", "rb");
FILE* fp3 = fopen("ciphertext3.dat", "rb");
FILE* fp4 = fopen("file.dat", "wb");
if (fp1 == NULL || fp2 == NULL || fp3 == NULL || fp4 == NULL) {
cout << "打开文件失败!" << endl;
return 0;
}
unsigned char* data = new unsigned char[blocksize];
unsigned char* plaintext = new unsigned char[blocksize];
while (!feof(fp1) || !feof(fp2) || !feof(fp3)) {
int len1 = fread(data, sizeof(unsigned char), blocksize, fp1);
int len2 = fread(data + len1, sizeof(unsigned char), blocksize - len1, fp2);
int len3 = fread(data + len1 + len2, sizeof(unsigned char), blocksize - len1 - len2, fp3);
int total = len1 + len2 + len3;
RC4(keydata, keylen, data, total, plaintext);
int len4 = fwrite(plaintext, sizeof(unsigned char), total, fp4);
if (len4 != total) {
cout << "写入文件失败!" << endl;
return 0;
}
}
fclose(fp1);
fclose(fp2);
fclose(fp3);
fclose(fp4);
delete[] data;
delete[] plaintext;
```
需要注意的是,文件分割和合并的操作只是示例代码,实际应用时需要更加完善和严密。
阅读全文
相关推荐
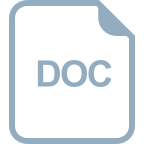
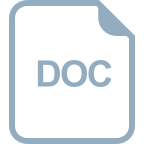
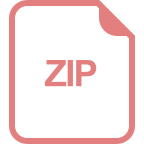
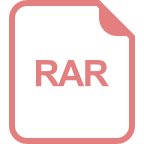
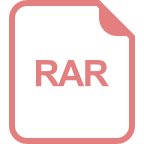
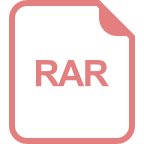
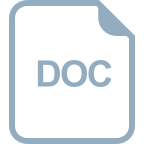
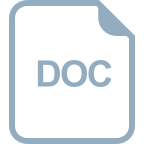
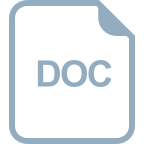
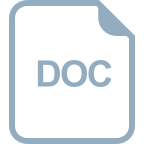
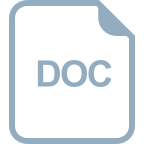




