c++实现 RC4加密算法
时间: 2023-10-27 19:06:39 浏览: 53
RC4加密算法是一种流密码算法,它可以用于数据的加密和解密。下面是一个用C语言实现RC4加密算法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
void swap(unsigned char *a, unsigned char *b) {
unsigned char temp = *a;
*a = *b;
*b = temp;
}
void rc4_init(unsigned char *key, int key_length, unsigned char S[256]) {
for (int i = 0; i < 256; i++) {
S[i] = i;
}
int j = 0;
for (int i = 0; i < 256; i++) {
j = (j + S[i] + key[i % key_length]) % 256;
swap(&S[i], &S[j]);
}
}
void rc4_crypt(unsigned char *input, unsigned char *output, int input_length, unsigned char S[256]) {
int i = 0;
int j = 0;
for (int k = 0; k < input_length; k++) {
i = (i + 1) % 256;
j = (j + S[i]) % 256;
swap(&S[i], &S[j]);
int t = (S[i] + S[j]) % 256;
output[k] = input[k] ^ S[t];
}
}
int main() {
unsigned char key[] = "ThisIsMyKey";
unsigned char plaintext[] = "Hello, world!";
int key_length = sizeof(key) - 1;
int plaintext_length = sizeof(plaintext) - 1;
unsigned char S[256];
rc4_init(key, key_length, S);
unsigned char ciphertext[plaintext_length];
rc4_crypt(plaintext, ciphertext, plaintext_length, S);
printf("Plaintext: %s\n", plaintext);
printf("Ciphertext: ");
for (int i = 0; i < plaintext_length; i++) {
printf("%02X ", ciphertext[i]);
}
printf("\n");
return 0;
}
```
在上面的示例代码中,`rc4_init`函数用于初始化RC4算法,`rc4_crypt`函数用于加密或解密数据。你可以直接运行这段代码,它将输出明文和加密后的密文。请注意,这只是一个简单的示例,实际使用时需要考虑密钥的生成和安全性等问题。
相关推荐
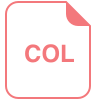
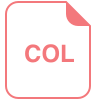
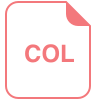
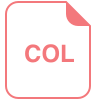
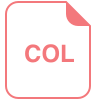









