用ECDSA加密算法生成csr,C++代码怎么写
时间: 2024-03-24 09:39:31 浏览: 123
以下是使用ECDSA加密算法生成CSR的C++代码示例:
```c++
#include <openssl/ec.h>
#include <openssl/err.h>
#include <openssl/evp.h>
#include <openssl/objects.h>
#include <openssl/pem.h>
#include <openssl/x509.h>
#define EC_CURVE_NAME NID_secp256k1 // 椭圆曲线名称
int main()
{
EVP_PKEY *pkey = NULL;
EC_KEY *eckey = NULL;
X509_REQ *req = NULL;
X509_NAME *subj = NULL;
int rc = 0;
// 创建 EC_KEY 对象
eckey = EC_KEY_new_by_curve_name(EC_CURVE_NAME);
if (eckey == NULL) {
fprintf(stderr, "EC_KEY_new_by_curve_name failed\n");
goto end;
}
// 生成密钥对
rc = EC_KEY_generate_key(eckey);
if (rc != 1) {
fprintf(stderr, "EC_KEY_generate_key failed\n");
goto end;
}
// 创建 X509_REQ 对象
req = X509_REQ_new();
if (req == NULL) {
fprintf(stderr, "X509_REQ_new failed\n");
goto end;
}
// 设置 subject 名称
subj = X509_REQ_get_subject_name(req);
rc = X509_NAME_add_entry_by_txt(subj, "CN", MBSTRING_ASC, (unsigned char *)"example.com", -1, -1, 0);
if (rc != 1) {
fprintf(stderr, "X509_NAME_add_entry_by_txt failed\n");
goto end;
}
// 将 EC_KEY 对象设置为 X509_REQ 对象的公钥
pkey = EVP_PKEY_new();
rc = EVP_PKEY_assign_EC_KEY(pkey, eckey);
if (rc != 1) {
fprintf(stderr, "EVP_PKEY_assign_EC_KEY failed\n");
goto end;
}
eckey = NULL; // 由 EVP_PKEY_free 负责释放
rc = X509_REQ_set_pubkey(req, pkey);
if (rc != 1) {
fprintf(stderr, "X509_REQ_set_pubkey failed\n");
goto end;
}
// 签名 X509_REQ 对象
rc = X509_REQ_sign(req, pkey, EVP_sha256());
if (rc == 0) {
fprintf(stderr, "X509_REQ_sign failed\n");
goto end;
}
// 将 X509_REQ 对象保存到文件中
FILE *fp = fopen("example.csr", "w");
if (fp == NULL) {
fprintf(stderr, "fopen failed\n");
goto end;
}
rc = PEM_write_X509_REQ(fp, req);
fclose(fp);
if (rc != 1) {
fprintf(stderr, "PEM_write_X509_REQ failed\n");
goto end;
}
end:
if (req != NULL) {
X509_REQ_free(req);
}
if (pkey != NULL) {
EVP_PKEY_free(pkey);
}
if (eckey != NULL) {
EC_KEY_free(eckey);
}
return rc;
}
```
该示例使用 OpenSSL 库,首先创建一个 EC_KEY 对象,使用该对象生成密钥对。然后创建 X509_REQ 对象,并设置主题名称。接着将 EC_KEY 对象设置为 X509_REQ 对象的公钥,并使用私钥对 X509_REQ 对象进行签名。最后将 X509_REQ 对象保存到文件中。
需要注意的是,该示例使用的椭圆曲线名称为 secp256k1,如果需要使用其他椭圆曲线,需要修改 EC_CURVE_NAME 的值。
阅读全文
相关推荐
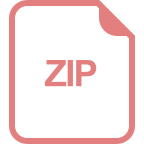
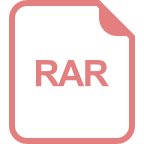
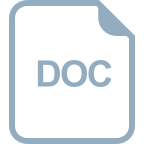
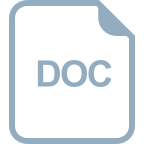
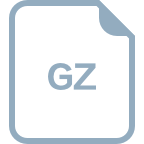
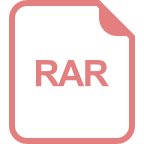
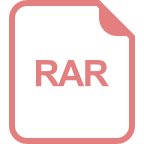
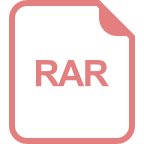
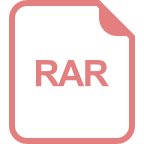
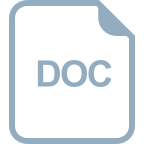
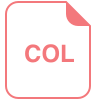







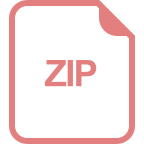