用C语言做一组代码,题目描述 你需要将一系列的学生期末考试信息进行存储(学生人数不会超过100)。每个学生的信息包括: 姓名(由 first name 和last name 两部分组成,例如Jingyu LI,first_name = "Jingyu" last_name = "LI") ; 学号(12 位数字组成,开头4位为2022、2021、2020); C语言成绩(一个大于等于零的整数); 重修信息(学号 2022.........为否,其余为是); GPA等级(A+, A, B+, B, C+, C, D, F )。 A+: 100-93; A: 92-85; B+: 84-80; B: 79-75; C+:74-70; C: 69-65; D: 64-60; F: <60. 其中,姓名,学号,成绩为输入数据,其余数据需要你计算。 另外,学号不符合规定的数据需要删除掉。 输入 一系列 名、姓、学号、成绩 输出 名、姓、学号、成绩、重修信息、GPA等级
时间: 2024-03-08 11:47:31 浏览: 160
下面是用C语言实现的代码:
```c
#include <stdio.h>
#include <string.h>
struct student {
char first_name[20];
char last_name[20];
char id[13];
int score;
int retake;
char grade[3];
};
int main() {
struct student stu[100];
int n, i, j = 0;
printf("请输入学生个数:");
scanf("%d", &n);
for(i = 0; i < n; i++) {
printf("请输入第%d个学生的信息:\n", i + 1);
scanf("%s %s %s %d", stu[j].first_name, stu[j].last_name, stu[j].id, &stu[j].score);
if(strncmp(stu[j].id, "2022", 4) != 0 && strncmp(stu[j].id, "2021", 4) != 0 && strncmp(stu[j].id, "2020", 4) != 0) {
printf("学号不符合规定,已删除该学生信息\n");
}
else {
if(stu[j].score < 0) {
stu[j].score = 0;
}
if(strncmp(stu[j].id, "2022", 4) == 0) {
stu[j].retake = 0;
}
else {
stu[j].retake = 1;
}
if(stu[j].score >= 93) {
strcpy(stu[j].grade, "A+");
}
else if(stu[j].score >= 85) {
strcpy(stu[j].grade, "A");
}
else if(stu[j].score >= 80) {
strcpy(stu[j].grade, "B+");
}
else if(stu[j].score >= 75) {
strcpy(stu[j].grade, "B");
}
else if(stu[j].score >= 70) {
strcpy(stu[j].grade, "C+");
}
else if(stu[j].score >= 65) {
strcpy(stu[j].grade, "C");
}
else if(stu[j].score >= 60) {
strcpy(stu[j].grade, "D");
}
else {
strcpy(stu[j].grade, "F");
}
j++;
}
}
printf("姓名\t学号\t\t成绩\t重修\tGPA等级\n");
for(i = 0; i < j; i++) {
printf("%s %s\t%s\t%d\t%s\t%s\n", stu[i].first_name, stu[i].last_name, stu[i].id, stu[i].score, stu[i].retake == 1 ? "是" : "否", stu[i].grade);
}
return 0;
}
```
代码中定义了一个结构体`student`,用来存储每个学生的信息,包括姓名、学号、成绩、重修信息和GPA等级。在主函数中,首先输入学生个数,然后循环读入每个学生的信息。读入信息后,先判断学号是否符合规定,如果不符合则删除该学生信息;如果符合,则计算重修信息和GPA等级,并将该学生信息保存到结构体数组中。最后输出所有学生的信息,包括姓名、学号、成绩、重修信息和GPA等级。
阅读全文
相关推荐
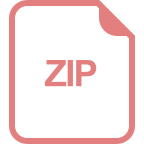
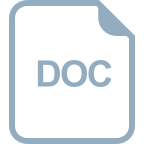
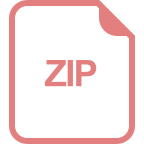
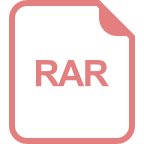
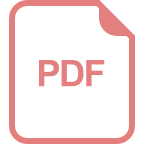
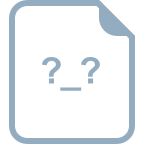
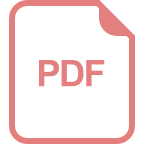
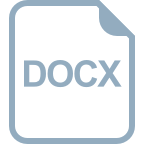
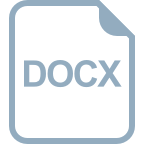
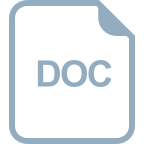
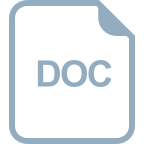
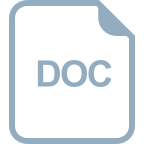
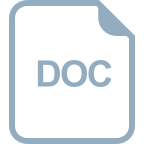
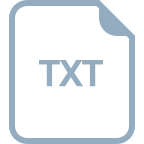
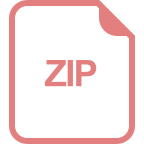
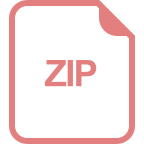
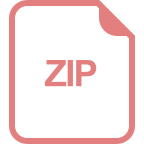
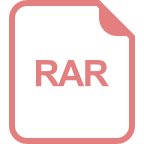