用c语言结构编写数据结构算法长整数的四则运算代码
时间: 2024-12-14 07:08:59 浏览: 18
在C语言中,为了实现长整数的四则运算,我们可以使用自定义的结构体来存储大整数,并利用数组来模拟每一位的数值。这里是一个基本的长整数加法的实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义长整数结构体
typedef struct {
int digit[100]; // 数组用于存储每一位数字
int length; // 记录当前表示的数字长度
} BigInt;
// 初始化函数
BigInt* createBigInt(int num) {
BigInt* bigInt = (BigInt*)malloc(sizeof(BigInt));
bigInt->length = 0;
while (num > 0) {
bigInt->digit[bigInt->length++] = num % 10;
num /= 10;
}
return bigInt;
}
// 尾部补零函数
void padZeroes(BigInt* bigInt) {
while (bigInt->length > 0 && bigInt->digit[bigInt->length - 1] == 0) {
bigInt->length--;
}
}
// 四则运算的核心部分:加法(这里仅展示加法)
BigInt* addBigints(BigInt* a, BigInt* b) {
BigInt* result = createBigInt(0);
int carry = 0;
for (int i = a->length - 1; i >= 0; i--) {
int sum = a->digit[i] + b->digit[i] + carry;
carry = sum / 10;
result->digit[result->length++] = sum % 10;
}
if (carry > 0) {
result->digit[result->length++] = carry;
}
padZeroes(result);
return result;
}
// 打印结果
void printBigInt(BigInt* bigInt) {
for (int i = bigInt->length - 1; i >= 0; i--) {
printf("%d", bigInt->digit[i]);
}
printf("\n");
}
int main() {
BigInt* a = createBigInt(45678);
BigInt* b = createBigInt(98765);
BigInt* sum = addBigints(a, b);
printBigInt(sum); // 输出结果
free(sum);
free(a);
free(b);
return 0;
}
```
这个例子只展示了加法,其他如减法、乘法和除法可以类似地实现。注意,在实际应用中,更高效的方式可能是使用字符串或动态内存管理,而不是数组。
阅读全文
相关推荐
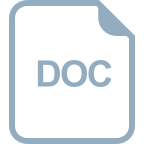
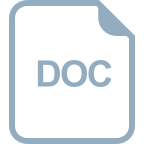
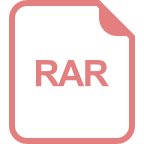
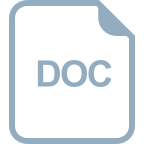
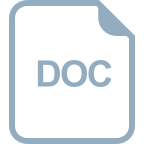
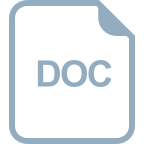

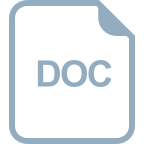
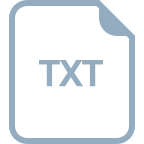
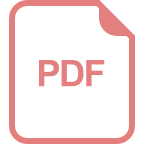
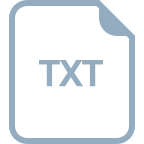
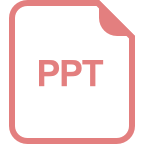
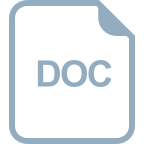
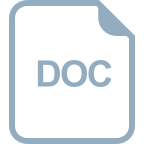
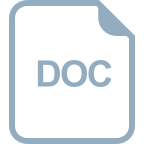
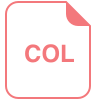
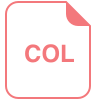
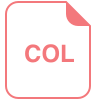