分别用单链表和双向链表编写关于交换相邻两个节点的C++代码
时间: 2024-09-21 11:12:44 浏览: 58
在C++中,我们可以分别为单链表和双向链表编写交换相邻两个节点的函数。这里提供简单的示例:
**对于单链表:**
```cpp
#include <iostream>
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(nullptr) {}
};
void swapAdjacentNodes(ListNode*& head) {
if (head == nullptr || head->next == nullptr)
return;
ListNode temp = *head;
ListNode* nextTemp = head->next;
head->next = nextTemp->next;
nextTemp->next = temp;
temp.next = nullptr; // 如果原头结点是新链表的第一个,需要置空
if (head->next != nullptr)
head->next->prev = head;
}
// 示例如何使用
int main() {
ListNode* list = new ListNode(1);
list->next = new ListNode(2);
list->next->next = new ListNode(3);
std::cout << "Before swap: ";
printList(list); // 自己实现一个打印链表的函数
swapAdjacentNodes(list);
std::cout << "After swap: ";
printList(list);
return 0;
}
```
**对于双向链表:**
```cpp
#include <iostream>
struct Node {
int val;
Node* prev;
Node* next;
Node(int x) : val(x), prev(nullptr), next(nullptr) {}
};
void swapAdjacentNodes(Node*& head) {
if (head == nullptr || head->next == nullptr)
return;
Node* temp = head->next;
head->next = temp->next;
temp->next->prev = head;
if (temp->next != nullptr)
temp->next->prev = temp;
else
head->prev = temp; // 确保旧头结点的前驱指向新尾结点
temp->prev = head->prev;
head->prev->next = temp;
}
// 示例如何使用
int main() {
Node* list = new Node(1);
list->prev = nullptr;
list->next = new Node(2);
list->next->prev = list;
list->next->next = new Node(3);
list->next->next->prev = list->next;
std::cout << "Before swap: ";
printList(list); // 自己实现一个打印链表的函数
swapAdjacentNodes(list);
std::cout << "After swap: ";
printList(list);
return 0;
}
```
请注意,`printList`函数需要你自己实现,用于遍历并打印链表。
阅读全文
相关推荐
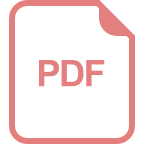
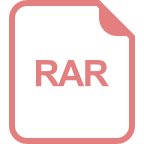
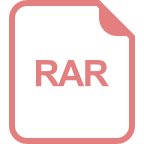
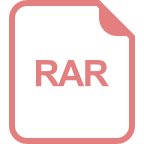
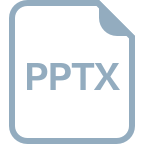
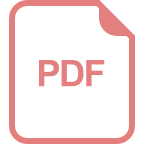
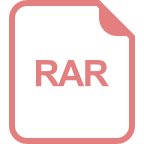
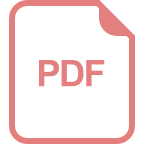
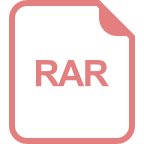







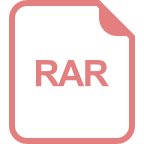
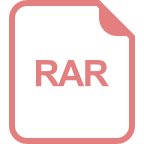
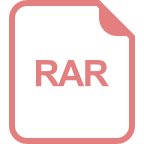