如何用C++实现双向链表中相邻节点的交换操作?
时间: 2024-11-07 13:13:57 浏览: 27
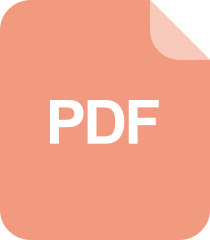
C++实现双向链表
在C++中,我们可以使用指针和迭代器来实现双向链表中相邻节点的交换操作。这里是一个简单的示例:
```cpp
#include <iostream>
// 定义双向链表节点结构体
struct Node {
int data;
Node* prev;
Node* next;
};
// 插入节点到链表的函数,便于后续操作
void insert(Node** head, int value) {
Node* newNode = new Node{value, nullptr, *head};
if (*head != nullptr)
(*head)->prev = newNode;
*head = newNode;
}
// 交换相邻节点的函数
void swapAdjacentNodes(Node** head) {
if (*head == nullptr || (*head)->next == nullptr)
return; // 链表为空或只有一个节点无需交换
Node* current = *head;
while (current->next != nullptr) {
std::swap(current->data, current->next->data);
current = current->next;
}
}
int main() {
// 创建并初始化双向链表
Node* head = nullptr;
insert(&head, 1);
insert(&head, 2);
insert(&head, 3);
insert(&head, 4);
std::cout << "Before swapping: ";
printList(head); // 假设printList函数用于打印链表
swapAdjacentNodes(&head);
std::cout << "After swapping: ";
printList(head);
return 0;
}
```
在这个例子中,`swapAdjacentNodes` 函数遍历链表,每次将当前节点的数据与下一个节点的数据交换。注意这仅适用于相邻节点的数据交换,如果需要任意两个节点之间的数据交换,你需要稍微调整一下算法。
阅读全文
相关推荐
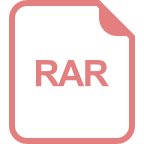
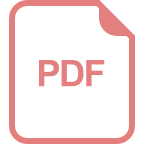

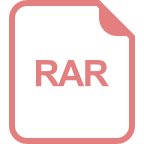
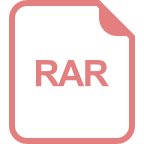
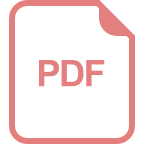
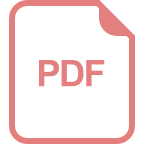
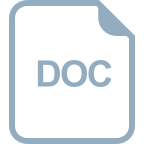
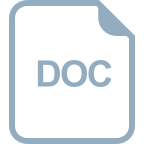

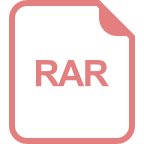
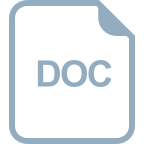
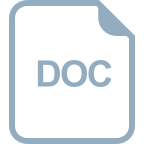
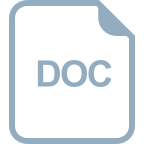

