计算一个英语句子里面有多少个单词并输出每个单词的个数和每一个单词,
时间: 2024-10-12 20:10:07 浏览: 74
计算英语句子中单词的数量以及它们的出现频率通常涉及到自然语言处理(NLP)技术。首先,需要对输入的句子进行分词,即将连续的字符序列分割成单个的词汇单元。这一步可能需要用到正则表达式、空格或其他特定的分隔符。
例如,在Python中,我们可以使用`nltk`库或者内置的split()函数:
```python
import re
sentence = "This is a sample sentence to demonstrate word counting."
words = re.findall(r'\b\w+\b', sentence.lower()) # 使用正则表达式分割并转换为小写
word_count = {word: words.count(word) for word in set(words)} # 统计每个单词的出现次数
for word, count in word_count.items():
print(f"Word '{word}': Count - {count}")
```
在这个例子中,我们先将所有单词转为小写并去除标点符号,然后统计每个单词的出现次数,并打印结果。
相关问题
计算一个英语句子里面有多少个单词并输出每个单词的个数和每一个单词,用java编写
在Java中,你可以使用`String`类的内置函数和一些字符串处理方法来计算一个英文句子中单词的数量,并逐个输出。以下是一个简单的示例:
```java
public class WordCount {
public static void main(String[] args) {
String sentence = "This is a sample English sentence with multiple words.";
// 分割句子为单词数组
String[] words = sentence.split("\\s+");
int wordCount = words.length;
System.out.println("Number of words in the sentence: " + wordCount);
for (int i = 0; i < wordCount; i++) {
System.out.println("Word " + (i+1) + ": " + words[i]);
}
}
}
```
在这个例子中,我们使用`split("\\s+")`方法将句子按照空格(包括空格、换行符等空白字符)分割成单词数组。然后,`words.length`得到单词总数,`for`循环遍历数组并打印每个单词及其索引。
输入一个英文句子,以回车结束,单词间以空格分隔,标点后跟一个空格,统计并输出单词的个数分行输出所有单词(不包括标点)。仅统计单词,数字按单词计,不计算标点符号,重复单词出现几次就统计几次
为了完成这个任务,我们可以使用Python编写一个小脚本。下面是一个简单的方法:
```python
import re
def count_words(sentence):
# 移除标点符号,并将字符串分割成单词列表
words = re.findall(r'\b\w+\b', sentence.strip())
# 创建一个字典来存储单词及其频率
word_count = {}
for word in words:
word_count[word] = word_count.get(word, 0) + 1
# 按照单词出现次数排序并打印结果
sorted_word_count = sorted(word_count.items(), key=lambda x: x[1], reverse=True)
for word, count in sorted_word_count:
print(f'{word}: {count}\n')
# 示例输入
sentence = input("请输入英文句子 (以回车结束): ")
count_words(sentence)
```
运行这个程序后,它会提示用户输入一个英文句子。然后,它会统计每个单词出现的次数,并按照出现频率从高到低打印出来。
阅读全文
相关推荐



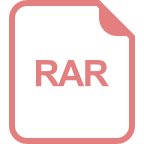












