提取文件中某一列内容c语言
时间: 2024-11-04 15:15:56 浏览: 30
在C语言中,提取文件中的某一列内容通常涉及到读取文本文件,并根据特定分隔符(比如逗号、制表符或空格)分割每一行的内容。这里提供一个简单的示例,假设你想从CSV文件中提取第二列(索引从0开始),并存储到一个新的数组中:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_LINE_LENGTH 100 // 文件行最大长度
#define COLUMN_SEPARATOR ',' // 列分字符
// 函数用于从文件中读取一行并解析指定列
void extract_column(const char *filename, int column_index, char *output[], int max_items) {
FILE *file = fopen(filename, "r");
if (file == NULL) {
perror("Failed to open file");
exit(1);
}
char line[MAX_LINE_LENGTH];
size_t line_length;
for (int i = 0; i < max_items && fgets(line, MAX_LINE_LENGTH, file); ++i) {
// 去除换行符
line[strlen(line) - 1] = '\0';
// 分割字符串
char *tokens = strtok(line, COLUMN_SEPARATOR);
while (tokens != NULL) {
if (column_index == 0) { // 如果是要提取第一列,直接赋值
output[i] = strdup(tokens);
} else {
// 对于其他列,跳过前面的列,直到找到目标列
for (size_t j = 0; j < column_index - 1; ++j) {
tokens = strtok(NULL, COLUMN_SEPARATOR);
}
output[i] = strdup(tokens + 1); // 加1是因为我们已经获取了第一个元素
}
tokens = strtok(NULL, COLUMN_SEPARATOR);
}
}
fclose(file);
}
// 示例用法
int main() {
char *data[10]; // 假设最多10条记录
int max_items = 10;
extract_column("example.csv", 1, data, max_items);
// 输出和处理提取的数据...
// ...
return 0;
}
```
注意,这个例子假设每行只有一个记录,且每个字段之间有固定的分隔符。实际应用中可能需要考虑更多复杂情况,例如处理缺失值、错误行等。
阅读全文
相关推荐
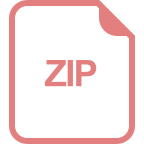
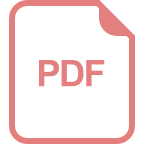
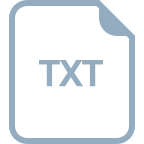


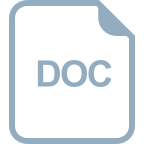
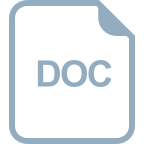
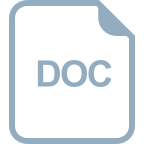
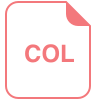
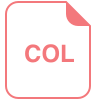








