python 程序写一个stable diffusion的提示词生成器
时间: 2023-08-16 13:10:27 浏览: 210
当然可以!下面是一个简单的Python程序示例,用于生成stable diffusion的提示词:
```python
import random
def generate_prompt():
prompts = [
"探索稳定扩散的机制",
"研究稳定扩散的特性",
"分析稳定扩散的影响因素",
"设计稳定扩散的模型",
"优化稳定扩散的算法",
"应用稳定扩散于实际问题",
"评估稳定扩散的效果"
]
return random.choice(prompts)
# 生成提示词
prompt = generate_prompt()
print(prompt)
```
这个程序定义了一个`generate_prompt`函数,其中包含了一些可能的提示词。通过调用`random.choice`函数,从提示词列表中随机选择一个提示词,并将其打印出来。
你可以根据需要修改提示词列表,并根据实际情况进行适当的调整。希望这个程序能够满足你的需求!
相关问题
用python语言编写一个使用stable diffusion模型生成图片的代码
好的!以下是一个使用Python编写的stable diffusion模型生成图片的代码示例:
```python
import numpy as np
from PIL import Image
# 定义stable diffusion函数
def stable_diffusion(image, alpha, delta_t, iterations):
# 获取图像的大小
height, width, channels = image.shape
# 初始化变量
u = np.zeros((height, width, channels))
u_new = np.zeros((height, width, channels))
# 将输入图像转换为浮点数类型
image = image.astype(np.float32)
# 初始化u和u_new
u[:] = image
u_new[:] = image
# 定义Laplacian算子
laplacian = np.array([[0, 1, 0], [1, -4, 1], [0, 1, 0]])
# 迭代stable diffusion模型
for i in range(iterations):
# 计算Laplacian of u
lu = np.zeros((height, width, channels))
for c in range(channels):
lu[:, :, c] = np.abs(np.fft.fftshift(np.fft.ifft2(np.fft.fft2(u[:, :, c]) * np.fft.fft2(laplacian, s=u.shape[:2], axes=(0, 1))))).real
# 更新u_new
u_new = u + delta_t * (lu ** alpha) * laplacian
# 更新u
u[:] = u_new
# 将u转换为整数类型
u = u.astype(np.uint8)
# 返回u
return u
# 加载图像
image = np.array(Image.open('input.jpg'))
# 运行stable diffusion模型
alpha = 1.0
delta_t = 0.1
iterations = 100
output_image = stable_diffusion(image, alpha, delta_t, iterations)
# 显示结果图像
Image.fromarray(output_image).show()
# 保存结果图像
Image.fromarray(output_image).save('output.jpg')
```
你可以将上述代码保存在一个.py文件中,然后使用Python解释器运行。请确保在代码中替换`input.jpg`和`output.jpg`为你所需的图像文件名。此外,你还可以尝试调整`alpha`,`delta_t`和`iterations`参数来获得不同的结果。
stable diffusion使用教程
Stable Diffusion 是一个用于模拟随机过程的 Python 库,它支持多种稳定分布。下面是 Stable Diffusion 的使用教程:
1. 安装 Stable Diffusion:
```python
pip install stable-baselines3[extra]
```
2. 导入 Stable Diffusion:
```python
from stable_baselines3.common.noise import StableNoise
```
3. 创建一个稳定噪声对象:
```python
noise = StableNoise(dim=1, sigma=0.1, alpha=1.5, beta=None, theta=None)
```
其中,`dim` 表示噪声的维度,`sigma` 表示噪声的标准差,`alpha` 表示稳定分布的 alpha 参数,`beta` 表示稳定分布的 beta 参数,`theta` 表示稳定分布的 theta 参数。
4. 生成一组随机数:
```python
noise.sample()
```
5. 可以使用 `reset` 方法重置随机数生成器:
```python
noise.reset()
```
6. 可以使用 `random_state` 参数指定随机种子:
```python
noise = StableNoise(dim=1, sigma=0.1, alpha=1.5, beta=None, theta=None, random_state=42)
```
以上就是 Stable Diffusion 的使用教程。希望对你有所帮助!
相关推荐
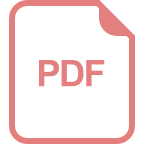







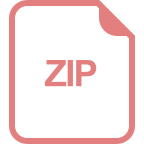
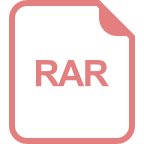
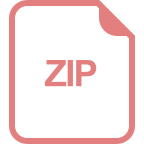
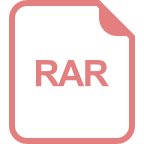
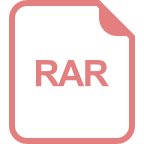
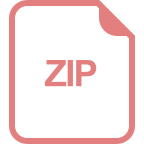