建立一个包含头节点和三个节点(4,2,1)的单链表,实现单链表建立的基本操作,完整C语言代码
时间: 2024-09-08 18:04:52 浏览: 69
在C语言中建立一个包含头节点和三个节点(值分别为4,2,1)的单链表,我们需要首先定义链表节点的数据结构,然后通过一系列的函数来创建和操作链表。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点的数据结构
typedef struct ListNode {
int val;
struct ListNode *next;
} ListNode;
// 创建一个新节点
ListNode* createNode(int val) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
if (!newNode) {
printf("内存分配失败\n");
return NULL;
}
newNode->val = val;
newNode->next = NULL;
return newNode;
}
// 初始化链表头节点
ListNode* initList() {
// 分配头节点并初始化,头节点的val通常不使用,所以可以设置为0或者其他值
ListNode* head = createNode(0);
if (head == NULL) {
return NULL;
}
return head;
}
// 在链表尾部插入新节点
void insertAtEnd(ListNode* head, int val) {
ListNode* newNode = createNode(val);
if (newNode == NULL) {
return;
}
// 寻找链表的最后一个节点
ListNode* current = head;
while (current->next != NULL) {
current = current->next;
}
// 插入新节点
current->next = newNode;
}
// 打印链表
void printList(ListNode* head) {
ListNode* current = head->next; // 跳过头节点
while (current != NULL) {
printf("%d ", current->val);
current = current->next;
}
printf("\n");
}
// 释放链表内存
void freeList(ListNode* head) {
ListNode* current = head;
while (current != NULL) {
ListNode* temp = current;
current = current->next;
free(temp);
}
}
int main() {
// 初始化链表
ListNode* list = initList();
if (list == NULL) {
return -1;
}
// 向链表中插入节点
insertAtEnd(list, 4);
insertAtEnd(list, 2);
insertAtEnd(list, 1);
// 打印链表
printf("单链表的内容为: ");
printList(list);
// 释放链表内存
freeList(list);
return 0;
}
```
这段代码首先定义了链表节点的结构体`ListNode`,接着定义了创建新节点的函数`createNode`,初始化链表的函数`initList`,在链表尾部插入新节点的函数`insertAtEnd`,打印链表的函数`printList`,以及释放链表内存的函数`freeList`。`main`函数中通过调用这些函数来创建链表并进行操作。
阅读全文
相关推荐
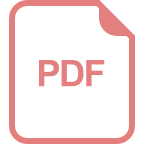
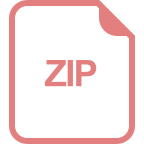
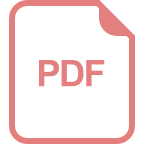
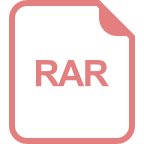
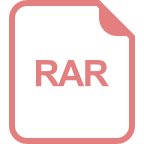
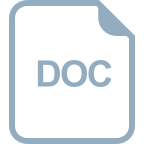
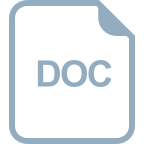
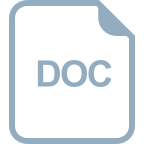
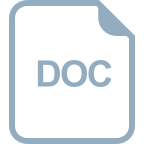
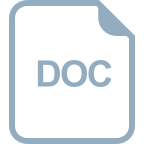
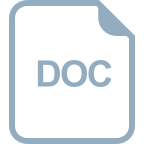






