使用skimage.filter模块的convolve2d函数
时间: 2024-12-10 10:48:35 浏览: 12
`skimage.filter`模块在Scikit-image库中提供了一个名为`convolve2d`的函数,专门用于二维数组(通常是图像)的卷积运算。这个函数接收两个参数:一个是输入的图像数组(源图像),另一个是卷积核(也称滤波器)。卷积核是一个小矩阵,它会滑动过整个图像并对每个位置执行元素乘法并求和,生成一个新的像素值。
以下是使用`convolve2d`的基本步骤:
```python
from skimage import io, filters
# 加载图像数据
img = io.imread('your_image.jpg', as_gray=True)
# 定义卷积核(这里是个简单的平均滤波器)
kernel = np.ones((3, 3)) / 9 # 3x3的均值滤波器
# 执行二维卷积
filtered_img = filters.convolve2d(img, kernel)
# 可能还需要调整输出尺寸,因为原始图像和滤波器可能不同大小
result = np.zeros_like(filtered_img)
result[:filtered_img.shape[0], :filtered_img.shape[1]] = filtered_img
io.imshow(result, cmap='gray') # 显示结果
```
在这个例子中,`as_gray=True`用于将彩色图像转换为灰度,以便于展示单通道的卷积效果。
相关问题
Traceback (most recent call last): File "E:\lastcode\TurbulenceSim_v1-master\Turbulence_Sim_v1_python\sample2.py", line 26, in <module> ssim = skimage.measure.compare_ssim(img1, img2, data_range=255) AttributeError: module 'skimage.measure' has no attribute 'compare_s
这个错误提示是因为你调用了`skimage.measure` 模块下的`compare_ssim` 函数,但这个函数在新版的scikit-image库中已经被移除了。因此,你需要使用其他的方法来计算结构相似性(SSIM)。
你可以通过以下方式计算SSIM:
1. 使用Scipy库中的`scipy.signal` 模块下的`convolve2d` 函数来计算高斯加权平均值。
2. 使用Scikit-Image库中的`skimage.filters` 模块下的`gaussian` 函数创建高斯滤波器。
3. 使用Scikit-Image库中的`skimage.metrics` 模块下的`structural_similarity` 函数计算结构相似性。
这里是一个示例代码:
```
from scipy.signal import convolve2d
from skimage.filters import gaussian
from skimage.metrics import structural_similarity
# Load two images
img1 = ...
img2 = ...
# Convert to grayscale if necessary
if img1.ndim == 3:
img1 = skimage.color.rgb2gray(img1)
if img2.ndim == 3:
img2 = skimage.color.rgb2gray(img2)
# Create Gaussian filter
sigma = 1.5
gaussian_filter = gaussian(11, sigma)
# Compute weighted means
mu1 = convolve2d(img1, gaussian_filter, mode='same')
mu2 = convolve2d(img2, gaussian_filter, mode='same')
# Compute weighted variances and covariances
sigma1_sq = convolve2d(np.power(img1 - mu1, 2), gaussian_filter, mode='same')
sigma2_sq = convolve2d(np.power(img2 - mu2, 2), gaussian_filter, mode='same')
sigma12 = convolve2d((img1 - mu1) * (img2 - mu2), gaussian_filter, mode='same')
# Compute SSIM
k1 = 0.01
k2 = 0.03
L = 255
C1 = np.power(k1 * L, 2)
C2 = np.power(k2 * L, 2)
ssim = structural_similarity(img1, img2, win_size=11, sigma=sigma, data_range=L, K1=C1, K2=C2)
```
这个示例代码中,我们首先加载了两个图像,然后将它们转换为灰度图像(如果它们是彩色图像)。接下来,我们创建了一个高斯滤波器,并用它计算了图像的加权均值和方差。最后,我们使用Scikit-Image库中的`structural_similarity` 函数计算了结构相似性。
convolve2d(image, kernel, 'same', boundary = 'fill', fillvalue = 0)怎么变成opencv
在OpenCV中,可以使用filter2D函数来实现二维卷积操作。该函数的使用方式如下:
```
cv2.filter2D(src, ddepth, kernel[, dst[, anchor[, delta[, borderType]]]])
```
其中,参数含义如下:
- src:输入图像。
- ddepth:输出图像的深度,通常为-1(与输入图像相同)。
- kernel:卷积核。
- dst:输出图像,可选参数。
- anchor:锚点,表示卷积核中心点的位置,默认为(-1,-1),即表示使用卷积核的中心点。
- delta:表示可选的偏置。
- borderType:表示边界填充方式,与convolve2d中的boundary参数对应,可选参数。
示例代码如下所示:
```
import cv2
import numpy as np
# 读取图像
img = cv2.imread('lena.jpg', cv2.IMREAD_GRAYSCALE)
# 定义卷积核
kernel = np.array([[1, 1, 1], [1, -8, 1], [1, 1, 1]])
# 使用filter2D函数进行卷积操作
dst = cv2.filter2D(img, -1, kernel, borderType=cv2.BORDER_CONSTANT)
# 显示结果
cv2.imshow('dst', dst)
cv2.waitKey()
cv2.destroyAllWindows()
```
在这个例子中,我们读取了一张灰度图像,并定义了一个3x3的卷积核,然后使用filter2D函数进行卷积操作,并将结果显示出来。
阅读全文
相关推荐
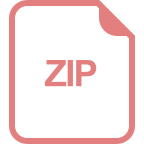
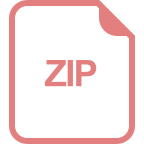
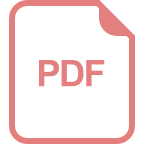
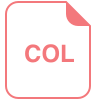
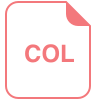
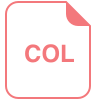
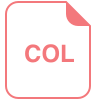
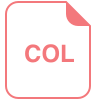







