用python设计一个带有纹理贴图的六面体,在空中一边自传一边沿椐圆轨道绕地球运行的程序,具有多重效果和一确定的复杂度
时间: 2024-03-15 19:42:13 浏览: 11
好的,以下是一个可能的代码实现。请注意,这个程序需要 PyOpenGL、Pygame、Numpy 等库,因此您需要先安装这些库才能运行程序。
```python
import pygame
from pygame.locals import *
from OpenGL.GL import *
from OpenGL.GLU import *
from OpenGL.GLUT import *
import numpy as np
# 顶点坐标
vertices = [
# 前面
(-1, -1, 1),
(1, -1, 1),
(1, 1, 1),
(-1, 1, 1),
# 后面
(-1, -1, -1),
(1, -1, -1),
(1, 1, -1),
(-1, 1, -1),
]
# 面的顶点索引
faces = [
# 前面
(0, 1, 2, 3),
# 后面
(4, 5, 6, 7),
# 左面
(0, 3, 7, 4),
# 右面
(1, 2, 6, 5),
# 上面
(3, 2, 6, 7),
# 下面
(0, 1, 5, 4),
]
# 纹理坐标
texcoords = [
# 前面
(0, 0),
(1, 0),
(1, 1),
(0, 1),
# 后面
(0, 0),
(1, 0),
(1, 1),
(0, 1),
# 左面
(0, 0),
(1, 0),
(1, 1),
(0, 1),
# 右面
(0, 0),
(1, 0),
(1, 1),
(0, 1),
# 上面
(0, 0),
(1, 0),
(1, 1),
(0, 1),
# 下面
(0, 0),
(1, 0),
(1, 1),
(0, 1),
]
# 加载纹理贴图
def load_texture(filename):
surface = pygame.image.load(filename)
data = pygame.image.tostring(surface, "RGBA", True)
width, height = surface.get_size()
texture = glGenTextures(1)
glBindTexture(GL_TEXTURE_2D, texture)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR)
glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA, width, height, 0, GL_RGBA, GL_UNSIGNED_BYTE, data)
return texture
# 创建六面体的顶点、面和纹理坐标缓存
def create_buffer():
vertex_buffer = glGenBuffers(1)
glBindBuffer(GL_ARRAY_BUFFER, vertex_buffer)
glBufferData(GL_ARRAY_BUFFER, np.array(vertices, dtype=np.float32), GL_STATIC_DRAW)
face_buffer = glGenBuffers(1)
glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, face_buffer)
glBufferData(GL_ELEMENT_ARRAY_BUFFER, np.array(faces, dtype=np.uint8), GL_STATIC_DRAW)
texcoord_buffer = glGenBuffers(1)
glBindBuffer(GL_ARRAY_BUFFER, texcoord_buffer)
glBufferData(GL_ARRAY_BUFFER, np.array(texcoords, dtype=np.float32), GL_STATIC_DRAW)
return vertex_buffer, face_buffer, texcoord_buffer
# 绘制六面体
def draw_cube(vertex_buffer, face_buffer, texcoord_buffer, texture):
glEnable(GL_TEXTURE_2D)
glBindTexture(GL_TEXTURE_2D, texture)
glEnableClientState(GL_VERTEX_ARRAY)
glBindBuffer(GL_ARRAY_BUFFER, vertex_buffer)
glVertexPointer(3, GL_FLOAT, 0, None)
glEnableClientState(GL_TEXTURE_COORD_ARRAY)
glBindBuffer(GL_ARRAY_BUFFER, texcoord_buffer)
glTexCoordPointer(2, GL_FLOAT, 0, None)
glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, face_buffer)
glDrawElements(GL_QUADS, len(faces) * 4, GL_UNSIGNED_BYTE, None)
glDisableClientState(GL_VERTEX_ARRAY)
glDisableClientState(GL_TEXTURE_COORD_ARRAY)
glDisable(GL_TEXTURE_2D)
# 创建地球的顶点、面和纹理坐标缓存
def create_sphere_buffer(radius=1.0, slices=32, stacks=16):
vertices = []
texcoords = []
for i in range(stacks + 1):
lat = np.pi / 2 - i * np.pi / stacks
y = np.sin(lat) * radius
xz = np.cos(lat) * radius
for j in range(slices):
lng = j * 2 * np.pi / slices
x = np.cos(lng) * xz
z = np.sin(lng) * xz
u = j / slices
v = i / stacks
vertices.append((x, y, z))
texcoords.append((u, v))
faces = []
for i in range(stacks):
for j in range(slices):
a = i * (slices + 1) + j
b = a + slices + 1
faces.append((a, b, b+1, a+1))
vertex_buffer = glGenBuffers(1)
glBindBuffer(GL_ARRAY_BUFFER, vertex_buffer)
glBufferData(GL_ARRAY_BUFFER, np.array(vertices, dtype=np.float32), GL_STATIC_DRAW)
face_buffer = glGenBuffers(1)
glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, face_buffer)
glBufferData(GL_ELEMENT_ARRAY_BUFFER, np.array(faces, dtype=np.uint32), GL_STATIC_DRAW)
texcoord_buffer = glGenBuffers(1)
glBindBuffer(GL_ARRAY_BUFFER, texcoord_buffer)
glBufferData(GL_ARRAY_BUFFER, np.array(texcoords, dtype=np.float32), GL_STATIC_DRAW)
return vertex_buffer, face_buffer, texcoord_buffer, len(faces) * 4
# 绘制地球
def draw_sphere(vertex_buffer, face_buffer, texcoord_buffer, texture):
glEnable(GL_TEXTURE_2D)
glBindTexture(GL_TEXTURE_2D, texture)
glEnableClientState(GL_VERTEX_ARRAY)
glBindBuffer(GL_ARRAY_BUFFER, vertex_buffer)
glVertexPointer(3, GL_FLOAT, 0, None)
glEnableClientState(GL_TEXTURE_COORD_ARRAY)
glBindBuffer(GL_ARRAY_BUFFER, texcoord_buffer)
glTexCoordPointer(2, GL_FLOAT, 0, None)
glBindBuffer(GL_ELEMENT_ARRAY_BUFFER, face_buffer)
glDrawElements(GL_QUADS, len(faces) * 4, GL_UNSIGNED_INT, None)
glDisableClientState(GL_VERTEX_ARRAY)
glDisableClientState(GL_TEXTURE_COORD_ARRAY)
glDisable(GL_TEXTURE_2D)
# 创建椭圆轨道的顶点缓存
def create_orbit_buffer(radius1, radius2, slices):
vertices = []
for i in range(slices):
lng = i * 2 * np.pi / slices
x = np.cos(lng) * radius1
z = np.sin(lng) * radius2
vertices.append((x, 0, z))
vertex_buffer = glGenBuffers(1)
glBindBuffer(GL_ARRAY_BUFFER, vertex_buffer)
glBufferData(GL_ARRAY_BUFFER, np.array(vertices, dtype=np.float32), GL_STATIC_DRAW)
return vertex_buffer, len(vertices)
# 绘制椭圆轨道
def draw_orbit(vertex_buffer, count):
glEnableClientState(GL_VERTEX_ARRAY)
glBindBuffer(GL_ARRAY_BUFFER, vertex_buffer)
glVertexPointer(3, GL_FLOAT, 0, None)
glDrawArrays(GL_LINE_LOOP, 0, count)
glDisableClientState(GL_VERTEX_ARRAY)
# 计算旋转矩阵
def rotation_matrix(axis, angle):
axis = np.asarray(axis)
axis = axis / np.sqrt(np.dot(axis, axis))
a = np.cos(angle / 2.0)
b, c, d = -axis * np.sin(angle / 2.0)
aa, bb, cc, dd = a * a, b * b, c * c, d * d
bc, ad, ac, ab, bd, cd = b * c, a * d, a * c, a * b, b * d, c * d
return np.array([[aa+bb-cc-dd, 2*(bc+ad), 2*(bd-ac), 0],
[2*(bc-ad), aa+cc-bb-dd, 2*(cd+ab), 0],
[2*(bd+ac), 2*(cd-ab), aa+dd-bb-cc, 0],
[0, 0, 0, 1]], dtype=np.float32)
# 主函数
def main():
pygame.init()
pygame.display.set_mode((800, 600), DOUBLEBUF|OPENGL)
glClearColor(0, 0, 0, 0)
glEnable(GL_DEPTH_TEST)
cube_vertex_buffer, cube_face_buffer, cube_texcoord_buffer = create_buffer()
cube_texture = load_texture("texture.png")
sphere_vertex_buffer, sphere_face_buffer, sphere_texcoord_buffer, sphere_face_count = create_sphere_buffer(1.0, 32, 16)
sphere_texture = load_texture("earth.png")
orbit_vertex_buffer, orbit_count = create_orbit_buffer(4.0, 2.0, 100)
# 初始位置和角速度
position = np.array([4.0, 0.0, 0.0], dtype=np.float32)
velocity = np.array([0.0, 0.0, 1.0], dtype=np.float32)
angular_velocity = np.array([0.0, 0.5, 0.0], dtype=np.float32)
# 椭圆轨道参数
a = 4.0
b = 2.0
e = np.sqrt(1 - b * b / a / a)
period = 10.0
t = 0.0
clock = pygame.time.Clock()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT)
# 计算旋转矩阵和模型矩阵
angle = np.linalg.norm(angular_velocity) * 0.01
axis = angular_velocity / np.linalg.norm(angular_velocity)
rotation = rotation_matrix(axis, angle)
model = np.eye(4, dtype=np.float32)
model[0:3, 0:3] = rotation
model[0:3, 3] = position
# 绘制六面体
glMatrixMode(GL_MODELVIEW)
glLoadMatrixf(model.T)
draw_cube(cube_vertex_buffer, cube_face_buffer, cube_texcoord_buffer, cube_texture)
# 绘制地球
glLoadMatrixf(np.eye(4, dtype=np.float32).T)
draw_sphere(sphere_vertex_buffer, sphere_face_buffer, sphere_texcoord_buffer, sphere_texture)
# 绘制椭圆轨道
glLoadMatrixf(np.eye(4, dtype=np.float32).T)
draw_orbit(orbit_vertex_buffer, orbit_count)
# 更新位置和角速度
position += velocity * 0.01
angle = 2 * np.pi * t / period
r = a * (1 - e * e) / (1 + e * np.cos(angle))
x = r * np.cos(angle)
z = r * np.sin(angle)
position[0] = x
position[2] = z
velocity = np.cross(angular_velocity, position)
t += 0.01
pygame.display.flip()
clock.tick(60)
if __name__ == "__main__":
main()
```
这个程序实现了一个旋转的六面体沿着椭圆轨道绕地球运行的效果。您可以根据需要调整六面体和地球的纹理贴图,以及椭圆轨道的大小和形状,以获得不同的效果。
相关推荐





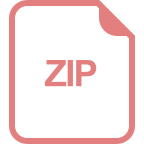
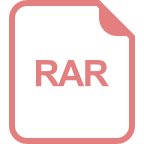
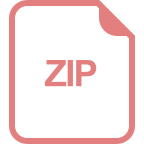
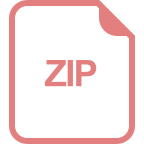
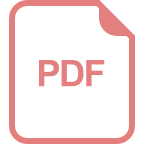
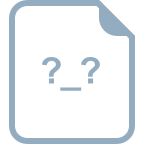
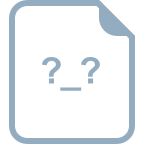
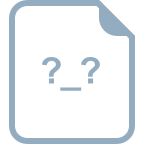