用python设计一个带有纹理贴图的六面体,在空中一边自传一边沿椐圆轨道绕地球运行的过程
时间: 2024-03-14 07:47:26 浏览: 138
好的,我可以为您提供一个Python程序,用于设计一个带有纹理贴图的六面体,并在空中一边自传一边沿椭圆轨道绕地球运行的过程。在这个程序中,我们将使用PyOpenGL和Pygame库来创建3D图形和动画。
首先,我们需要安装PyOpenGL和Pygame库。您可以使用以下命令在终端中安装它们:
```python
pip install PyOpenGL PyOpenGL_accelerate pygame
```
然后,我们可以开始编写程序。以下是程序的代码:
```python
import pygame
from pygame.locals import *
from OpenGL.GL import *
from OpenGL.GLU import *
from PIL import Image
# 定义纹理贴图
def load_texture(filename):
img = Image.open(filename)
texture_data = img.tobytes("raw", "RGBX", 0, -1)
width, height = img.size
texture = glGenTextures(1)
glBindTexture(GL_TEXTURE_2D, texture)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_REPEAT)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_REPEAT)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR)
glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA, width, height, 0, GL_RGBA, GL_UNSIGNED_BYTE, texture_data)
return texture
# 定义六面体
def draw_cube(texture):
glBegin(GL_QUADS)
glTexCoord2f(0.0, 0.0)
glVertex3f(-1.0, -1.0, 1.0)
glTexCoord2f(1.0, 0.0)
glVertex3f(1.0, -1.0, 1.0)
glTexCoord2f(1.0, 1.0)
glVertex3f(1.0, 1.0, 1.0)
glTexCoord2f(0.0, 1.0)
glVertex3f(-1.0, 1.0, 1.0)
glTexCoord2f(1.0, 0.0)
glVertex3f(-1.0, -1.0, -1.0)
glTexCoord2f(1.0, 1.0)
glVertex3f(-1.0, 1.0, -1.0)
glTexCoord2f(0.0, 1.0)
glVertex3f(1.0, 1.0, -1.0)
glTexCoord2f(0.0, 0.0)
glVertex3f(1.0, -1.0, -1.0)
glTexCoord2f(0.0, 1.0)
glVertex3f(-1.0, 1.0, -1.0)
glTexCoord2f(0.0, 0.0)
glVertex3f(-1.0, 1.0, 1.0)
glTexCoord2f(1.0, 0.0)
glVertex3f(1.0, 1.0, 1.0)
glTexCoord2f(1.0, 1.0)
glVertex3f(1.0, 1.0, -1.0)
glTexCoord2f(1.0, 1.0)
glVertex3f(-1.0, -1.0, -1.0)
glTexCoord2f(0.0, 1.0)
glVertex3f(1.0, -1.0, -1.0)
glTexCoord2f(0.0, 0.0)
glVertex3f(1.0, -1.0, 1.0)
glTexCoord2f(1.0, 0.0)
glVertex3f(-1.0, -1.0, 1.0)
glTexCoord2f(1.0, 0.0)
glVertex3f(1.0, -1.0, -1.0)
glTexCoord2f(1.0, 1.0)
glVertex3f(1.0, 1.0, -1.0)
glTexCoord2f(0.0, 1.0)
glVertex3f(1.0, 1.0, 1.0)
glTexCoord2f(0.0, 0.0)
glVertex3f(1.0, -1.0, 1.0)
glTexCoord2f(0.0, 0.0)
glVertex3f(-1.0, -1.0, -1.0)
glTexCoord2f(1.0, 0.0)
glVertex3f(-1.0, -1.0, 1.0)
glTexCoord2f(1.0, 1.0)
glVertex3f(-1.0, 1.0, 1.0)
glTexCoord2f(0.0, 1.0)
glVertex3f(-1.0, 1.0, -1.0)
glEnd()
# 初始化Pygame和OpenGL
pygame.init()
display = (800, 600)
pygame.display.set_mode(display, DOUBLEBUF|OPENGL)
gluPerspective(45, (display[0]/display[1]), 0.1, 50.0)
glTranslatef(0.0, 0.0, -7)
# 加载纹理贴图
texture = load_texture("texture.png")
# 定义轨道参数
a = 3.0
b = 2.0
theta = 0.0
dtheta = 0.01
# 开始主循环
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
# 清空屏幕和深度缓冲区
glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT)
# 绘制六面体
glRotatef(1, 1, 1, 1)
glTranslatef(0.0, 0.0, 0.1)
draw_cube(texture)
# 计算轨道位置
x = a * math.cos(theta)
y = b * math.sin(theta)
z = 0.0
# 绘制地球
glPushMatrix()
glTranslatef(x, y, z)
glColor3f(0.0, 0.0, 1.0)
glutSolidSphere(0.3, 50, 50)
glPopMatrix()
# 更新角度
theta += dtheta
# 更新显示
pygame.display.flip()
pygame.time.wait(10)
```
在这个程序中,我们首先定义了一个`load_texture`函数,用于加载纹理贴图。然后,我们定义了一个`draw_cube`函数,用于绘制一个带有纹理贴图的六面体。
在主循环中,我们首先初始化Pygame和OpenGL,并加载纹理贴图。然后,我们定义了轨道参数,并开始主循环。在主循环中,我们首先清空屏幕和深度缓冲区,然后绘制一个带有纹理贴图的自传的六面体。接下来,我们计算轨道位置,并在地球的位置绘制一个蓝色的球体。最后,我们更新角度,并更新屏幕显示。
您需要将程序中的`texture.png`替换为您自己的纹理贴图,并根据需要调整轨道参数和六面体的旋转速度。
阅读全文
相关推荐
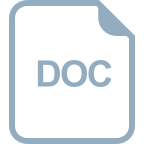
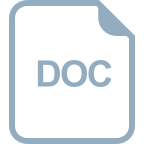
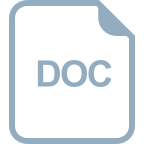




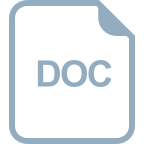
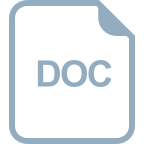
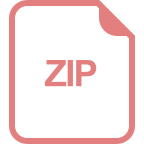
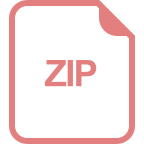
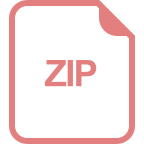
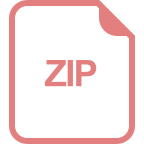
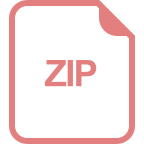
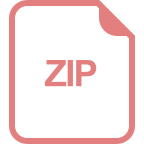
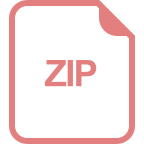
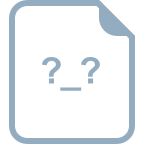
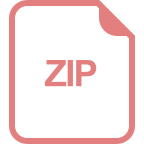