3D坐标系内绘制一个球体
时间: 2024-05-14 19:17:14 浏览: 124
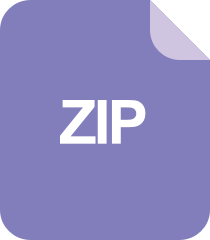
3D视觉效果的球体
要在3D坐标系内绘制一个球体,可以使用以下步骤:
1. 定义球体的半径r和中心点坐标(x0, y0, z0)。
2. 确定球体的绘制精度,例如可以设置经度和纬度的步长(step)。
3. 使用三重循环遍历经度、纬度,计算每个点的坐标,并将其加入顶点列表中。
4. 使用OpenGL或其他3D图形库,根据顶点列表绘制球体。
以下是示例代码,仅供参考:
```python
import math
from OpenGL.GL import *
def draw_sphere(x0, y0, z0, r, step):
vertices = []
for i in range(-90, 90, step):
lat1 = math.radians(i)
lat2 = math.radians(i + step)
for j in range(0, 360, step):
lon1 = math.radians(j)
lon2 = math.radians(j + step)
x1 = r * math.cos(lat1) * math.cos(lon1) + x0
y1 = r * math.cos(lat1) * math.sin(lon1) + y0
z1 = r * math.sin(lat1) + z0
x2 = r * math.cos(lat1) * math.cos(lon2) + x0
y2 = r * math.cos(lat1) * math.sin(lon2) + y0
z2 = r * math.sin(lat1) + z0
x3 = r * math.cos(lat2) * math.cos(lon2) + x0
y3 = r * math.cos(lat2) * math.sin(lon2) + y0
z3 = r * math.sin(lat2) + z0
x4 = r * math.cos(lat2) * math.cos(lon1) + x0
y4 = r * math.cos(lat2) * math.sin(lon1) + y0
z4 = r * math.sin(lat2) + z0
vertices.extend([x1, y1, z1, x2, y2, z2, x3, y3, z3, x1, y1, z1, x3, y3, z3, x4, y4, z4])
glBegin(GL_TRIANGLES)
for i in range(0, len(vertices), 3):
glVertex3fv(vertices[i:i+3])
glEnd()
```
阅读全文
相关推荐

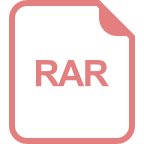
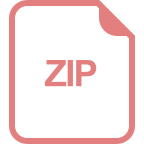
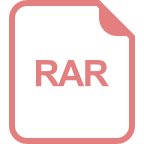
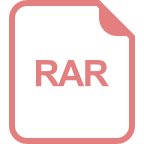
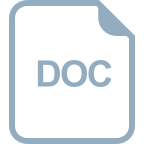
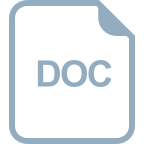
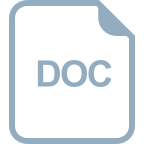
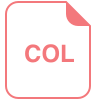
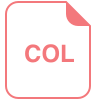





