Java中数组可以作为hashmap的键吗?
时间: 2023-06-15 21:03:34 浏览: 95
在Java中,数组可以作为HashMap的键,但是需要注意一些细节。数组作为HashMap的键时,需要满足以下条件:
1. 数组必须重写hashCode()和equals()方法。
2. 数组作为键时,只有当数组的内容完全相同才会被认为是同一个键。
3. 如果数组作为键的话,数组的长度也会被考虑在内,即不同长度的数组即使内容相同也被认为是不同的键。
因为数组的长度会对hashCode()方法的返回值产生影响,所以需要在计算hashCode()时同时考虑数组的长度。另外,在equals()方法中也需要比较数组的长度。下面是一个示例代码:
```
import java.util.HashMap;
public class ArrayAsKey {
public static void main(String[] args) {
int[] arr1 = {1, 2, 3};
int[] arr2 = {1, 2, 3};
int[] arr3 = {1, 2, 3, 4};
HashMap<int[], String> map = new HashMap<>();
map.put(arr1, "value1");
map.put(arr2, "value2");
map.put(arr3, "value3");
System.out.println(map.get(arr1)); // value2
System.out.println(map.get(arr2)); // value2
System.out.println(map.get(arr3)); // value3
}
@Override
public int hashCode() {
int result = 17;
for (int i = 0; i < this.length; i++) {
result = 31 * result + this[i];
}
result = 31 * result + this.length;
return result;
}
@Override
public boolean equals(Object other) {
if (!(other instanceof int[])) {
return false;
}
int[] otherArray = (int[]) other;
if (this.length != otherArray.length) {
return false;
}
for (int i = 0; i < this.length; i++) {
if (this[i] != otherArray[i]) {
return false;
}
}
return true;
}
}
```
阅读全文
相关推荐
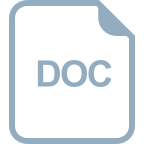
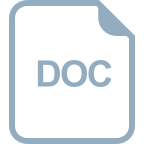
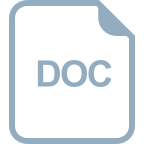
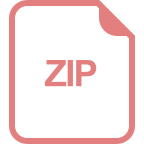
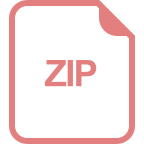


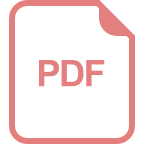
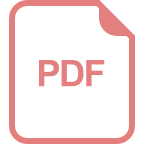
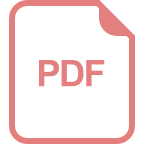
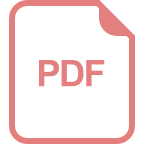
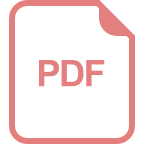
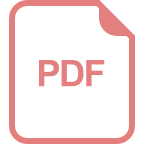
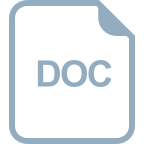
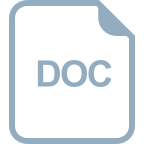
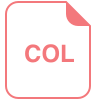
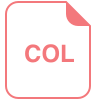

