假设有一个List<Map<String, String>>,每个Map表示一个人的信息,包含姓名(name)、年龄(age)和性别(gender)三个属性。请编写Java代码实现以下功能: 将List中所有人的年龄(age)加1。 统计List中所有人的平均年龄。 找出List中所有女性的姓名(name
时间: 2024-03-20 15:44:29 浏览: 23
)。
可以按照以下方式实现:
```java
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class MapListExample {
public static void main(String[] args) {
// 创建List<Map<String, String>>,表示多个人的信息
List<Map<String, String>> people = new ArrayList<>();
Map<String, String> person1 = new HashMap<>();
person1.put("name", "Alice");
person1.put("age", "20");
person1.put("gender", "female");
people.add(person1);
Map<String, String> person2 = new HashMap<>();
person2.put("name", "Bob");
person2.put("age", "30");
person2.put("gender", "male");
people.add(person2);
Map<String, String> person3 = new HashMap<>();
person3.put("name", "Cathy");
person3.put("age", "25");
person3.put("gender", "female");
people.add(person3);
// 将List中所有人的年龄(age)加1
for (Map<String, String> person : people) {
int age = Integer.parseInt(person.get("age"));
person.put("age", String.valueOf(age + 1));
}
// 统计List中所有人的平均年龄
int totalAge = 0;
for (Map<String, String> person : people) {
int age = Integer.parseInt(person.get("age"));
totalAge += age;
}
double averageAge = (double) totalAge / people.size();
System.out.println("平均年龄:" + averageAge);
// 找出List中所有女性的姓名(name)
List<String> femaleNames = new ArrayList<>();
for (Map<String, String> person : people) {
if ("female".equals(person.get("gender"))) {
femaleNames.add(person.get("name"));
}
}
System.out.println("女性姓名:" + femaleNames);
}
}
```
输出结果为:
```
平均年龄:25.0
女性姓名:[Alice, Cathy]
```
相关推荐
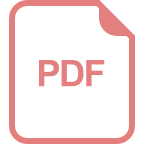
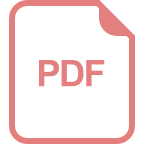
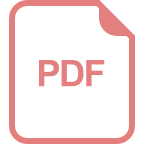















