js实现自动轮播走马灯,并且能左右手动控制
时间: 2024-01-20 11:03:45 浏览: 89
下面是一个简单的实现自动轮播的走马灯,并且能够左右手动控制的 JavaScript 代码:
HTML代码:
```html
<div class="carousel">
<div class="carousel-inner">
<div class="carousel-item active">
<img src="image1.jpg" alt="">
</div>
<div class="carousel-item">
<img src="image2.jpg" alt="">
</div>
<div class="carousel-item">
<img src="image3.jpg" alt="">
</div>
</div>
<a class="carousel-control prev"></a>
<a class="carousel-control next"></a>
</div>
```
CSS代码:
```css
.carousel {
position: relative;
}
.carousel-inner {
position: relative;
overflow: hidden;
}
.carousel-item {
position: absolute;
top: 0;
left: 0;
right: 0;
bottom: 0;
opacity: 0;
transition: opacity 1s ease-in-out;
}
.carousel-item.active {
opacity: 1;
}
.carousel-control {
position: absolute;
top: 50%;
transform: translateY(-50%);
width: 50px;
height: 50px;
background-color: rgba(0, 0, 0, 0.5);
border-radius: 100%;
color: #fff;
text-align: center;
font-size: 24px;
line-height: 50px;
cursor: pointer;
}
.carousel-control.prev {
left: 10px;
}
.carousel-control.next {
right: 10px;
}
```
JavaScript代码:
```javascript
var carousel = document.querySelector('.carousel');
var carouselInner = carousel.querySelector('.carousel-inner');
var carouselItems = carousel.querySelectorAll('.carousel-item');
var prevButton = carousel.querySelector('.carousel-control.prev');
var nextButton = carousel.querySelector('.carousel-control.next');
var currentIndex = 0;
var intervalId;
function showImage(index) {
carouselItems[currentIndex].classList.remove('active');
carouselItems[index].classList.add('active');
currentIndex = index;
}
function startAutoPlay() {
intervalId = setInterval(function() {
var nextIndex = currentIndex + 1;
if (nextIndex >= carouselItems.length) {
nextIndex = 0;
}
showImage(nextIndex);
}, 3000);
}
function stopAutoPlay() {
clearInterval(intervalId);
}
startAutoPlay();
prevButton.addEventListener('click', function() {
var prevIndex = currentIndex - 1;
if (prevIndex < 0) {
prevIndex = carouselItems.length - 1;
}
stopAutoPlay();
showImage(prevIndex);
});
nextButton.addEventListener('click', function() {
var nextIndex = currentIndex + 1;
if (nextIndex >= carouselItems.length) {
nextIndex = 0;
}
stopAutoPlay();
showImage(nextIndex);
});
carousel.addEventListener('mouseover', stopAutoPlay);
carousel.addEventListener('mouseout', startAutoPlay);
```
解释:
1. 首先获取需要的元素,包括走马灯容器、轮播图列表、左右切换按钮等。
2. 定义一个变量 `currentIndex` 来记录当前轮播图的索引,初始值为 0。
3. 定义一个函数 `showImage`,用来显示指定索引的轮播图。它会将当前轮播图的 `active` 类移除,将指定索引的轮播图添加 `active` 类,并更新 `currentIndex` 的值。
4. 定义一个函数 `startAutoPlay`,用来开始自动轮播。它使用 `setInterval` 函数来定时进行轮播,每隔 3 秒钟切换到下一张轮播图。
5. 定义一个函数 `stopAutoPlay`,用来停止自动轮播。它使用 `clearInterval` 函数来清除自动轮播的定时器。
6. 调用 `startAutoPlay` 函数,开始自动轮播。
7. 给左右切换按钮分别添加点击事件监听器,用来手动切换轮播图。当点击左切换按钮时,会显示上一张轮播图;当点击右切换按钮时,会显示下一张轮播图。
8. 给走马灯容器分别添加鼠标移入和移出事件监听器,用来在鼠标悬停时停止自动轮播,在鼠标离开时恢复自动轮播。
阅读全文
相关推荐
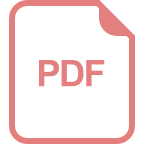
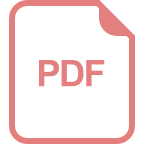

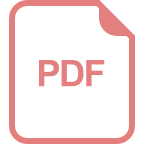
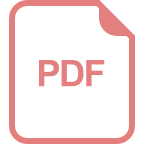
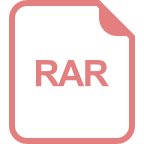
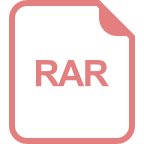
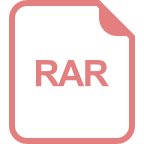
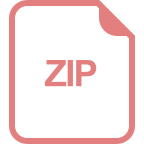
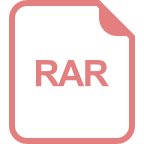
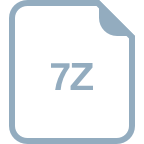
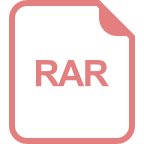
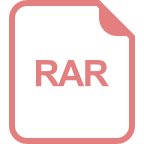
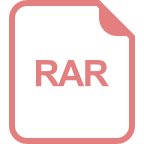
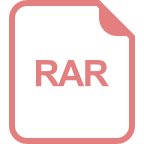
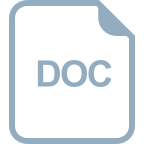

