如何实现将一个batchsize的数据平分给两个GPU同时训练,模型的权重参数同步更新
时间: 2024-10-24 18:14:12 浏览: 25
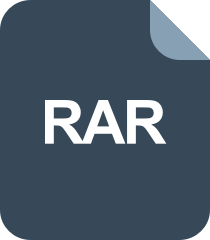
灰狼算法优化LSTM超参数-神经元个数-dropout-batch-size

要实现将一个 batch size 的数据平分给两个 GPU 并同步更新模型权重参数,通常采用 PyTorch 中的 DistributedDataParallel (DDP) 或者 Horovod 等分布式训练库。这里简单概述过程:
1. **设置环境**:
- 安装必要的库,如 torch.distributed 和 torch.nn.parallel.DistributedDataParallel。
2. **初始化分布式**:
```python
import torch.distributed as dist
from torch.nn.parallel import DistributedDataParallel as DDP
if not dist.is_available():
raise Exception("Distributed training requires torch distributed to be available.")
if not dist.is_initialized():
# 初始化分布式进程组
world_size = 2 # 根据实际GPU数量设定
init_method = "tcp://localhost:12345" # 使用适合的初始化方法
dist.init_process_group(backend="nccl", init_method=init_method, rank=torch.cuda.current_device(), world_size=world_size)
```
3. **创建模型并转换为分布式**:
```python
model = YourModel() # 创建模型实例
model = DDP(model) # 转换为分布式模型
```
4. **数据分割**:
- 在每个GPU上接收不同的部分数据。
```python
def get_data_loader_per_gpu(batch_size):
local_batch_size = batch_size // world_size
# 创建数据加载器,按照每个GPU分配的数据量
data_loaders = [...]
return data_loaders
train_dataloader = get_data_loader_per_gpu(batch_size)
```
5. **训练循环**:
- 在每个迭代中,两个GPU并行执行 forward, backward, 和 optimizer.step()。
```python
for inputs in zip(*train_dataloaders): # 数据集拆分到多个进程
with torch.no_grad():
outputs = model(inputs[0].cuda()) # 将数据移动到当前GPU
loss = ... # 计算损失
loss.backward()
# 梯度同步(在主GPU上)
if torch.distributed.get_rank() == 0:
torch.distributed.all_reduce(loss) # 减少损失
optimizer.step() # 更新参数
optimizer.zero_grad()
```
6. **关闭分布式**:
```python
if dist.is_initialized():
dist.destroy_process_group()
```
阅读全文
相关推荐
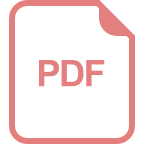
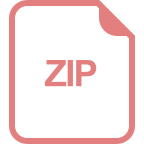
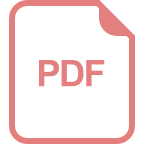
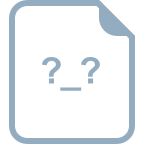
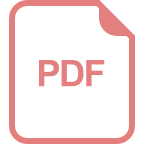
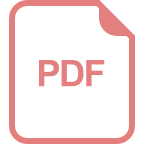
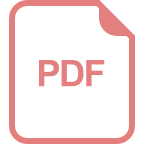
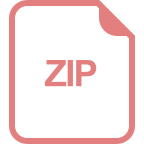
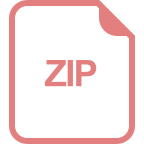
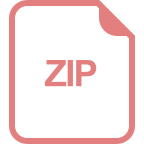
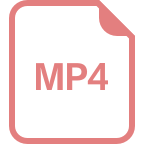
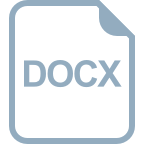
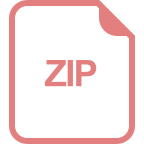
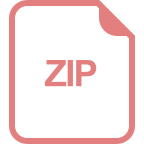
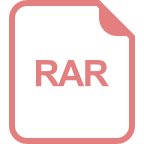