数据结构二叉排序树查找代码
时间: 2023-07-25 15:15:19 浏览: 112
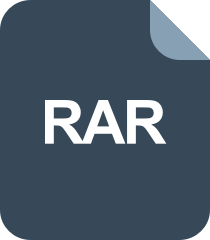
数据结构二叉树代码

下面是一个简单的二叉排序树查找的代码实现,仅供参考:
```c
// 定义二叉排序树结点
typedef struct BSTNode {
int key; // 关键字
struct BSTNode *left; // 左子节点
struct BSTNode *right; // 右子节点
} BSTNode;
// 插入一个结点到二叉排序树中
void insertBSTNode(BSTNode **root, int key) {
if (*root == NULL) { // 如果根节点为空,则创建新结点
BSTNode *newNode = (BSTNode*)malloc(sizeof(BSTNode));
newNode->key = key;
newNode->left = NULL;
newNode->right = NULL;
*root = newNode;
} else { // 根节点不为空,则插入到左子树或右子树中
if (key < (*root)->key) { // 插入到左子树中
insertBSTNode(&(*root)->left, key);
} else if (key > (*root)->key) { // 插入到右子树中
insertBSTNode(&(*root)->right, key);
}
}
}
// 在二叉排序树中查找指定关键字的结点
BSTNode* searchBSTNode(BSTNode *root, int key) {
if (root == NULL) { // 未找到结点
return NULL;
} else if (root->key == key) { // 找到结点
return root;
} else if (key < root->key) { // 在左子树中查找
return searchBSTNode(root->left, key);
} else { // 在右子树中查找
return searchBSTNode(root->right, key);
}
}
```
使用时,可以先创建一个空的二叉排序树,然后逐个插入结点,最后在树中查找指定关键字的结点。例如:
```c
int main() {
BSTNode *root = NULL;
// 插入结点
insertBSTNode(&root, 8);
insertBSTNode(&root, 3);
insertBSTNode(&root, 10);
insertBSTNode(&root, 1);
insertBSTNode(&root, 6);
insertBSTNode(&root, 14);
insertBSTNode(&root, 4);
insertBSTNode(&root, 7);
insertBSTNode(&root, 13);
// 查找结点
BSTNode *node = searchBSTNode(root, 6);
if (node != NULL) {
printf("Found node: %d\n", node->key);
} else {
printf("Node not found.\n");
}
return 0;
}
```
阅读全文
相关推荐
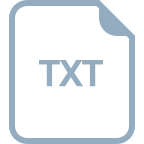
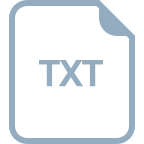
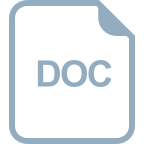
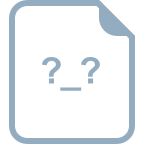
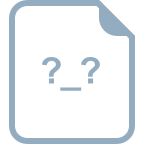
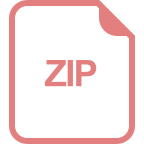
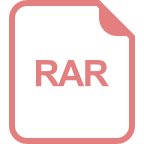
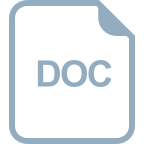
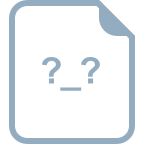
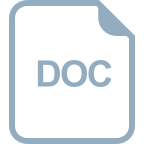
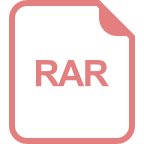
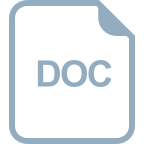
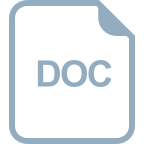
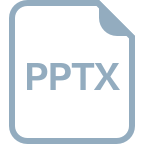
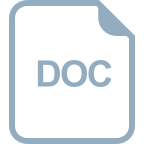