用Java语言实现记事本中的拼写检查
时间: 2023-09-15 15:16:13 浏览: 62
要实现记事本中的拼写检查,可以通过以下步骤来实现:
1. 获取用户输入的文本内容。
2. 将文本内容分割为单词,可以使用 Java 自带的 String 类的 split 方法,指定分隔符为空格。
3. 针对每个单词,使用 Java 自带的 SpellChecker 类中的方法进行拼写检查。可以使用 Jazzy 提供的 SpellChecker 类来实现,这个类提供了多种不同的语言的拼写检查器。
4. 对于拼写错误的单词,可以使用 Java 自带的 JOptionPane 类来弹出提示框,提示用户进行修改。
下面是一个简单的示例代码,可以实现基本的拼写检查功能:
```java
import java.util.Locale;
import java.util.Scanner;
import javax.swing.JOptionPane;
import com.swabunga.spell.engine.SpellDictionaryHashMap;
import com.swabunga.spell.event.SpellChecker;
import com.swabunga.spell.event.StringWordTokenizer;
public class SpellCheckDemo {
public static void main(String[] args) {
// 加载英文拼写词典
SpellDictionaryHashMap dictionary = new SpellDictionaryHashMap(
new Scanner(SpellCheckDemo.class.getResourceAsStream("/dictionary/english.0")));
SpellChecker spellChecker = new SpellChecker(dictionary);
// 获取用户输入的文本内容
String text = JOptionPane.showInputDialog(null, "请输入文本内容:");
// 将文本内容分割为单词
String[] words = text.split("\\s+");
// 针对每个单词进行拼写检查
for (String word : words) {
if (!spellChecker.isCorrect(new StringWordTokenizer(word))) {
// 如果拼写错误,弹出提示框
JOptionPane.showMessageDialog(null, "拼写错误:" + word);
}
}
}
}
```
注意,这个示例代码只实现了基本的拼写检查功能,实际使用中还需要考虑更多的细节问题,比如如何处理标点符号、如何处理数字等等。
相关推荐
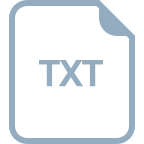
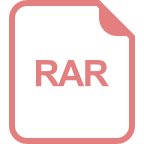
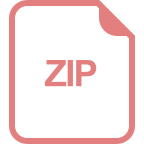














