动态链表生成算法思路C语言
时间: 2023-10-12 12:12:56 浏览: 95
动态链表是一种常见的数据结构,它的基本操作包括插入、删除、查找等。下面是一个简单的动态链表生成算法思路,使用C语言实现。
1. 定义一个结构体来表示链表中的一个节点,包含数据和指向下一个节点的指针:
```c
struct Node {
int data;
struct Node* next;
};
```
2. 定义一个指向链表头结点的指针,并初始化为NULL:
```c
struct Node* head = NULL;
```
3. 定义一个循环,用于输入数据并插入到链表中。循环的退出条件可以是输入一个特定的值,比如-1:
```c
while (1) {
int value;
printf("Enter a value (-1 to exit): ");
scanf("%d", &value);
if (value == -1) {
break;
}
// create a new node
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = value;
newNode->next = NULL;
// insert the new node into the list
if (head == NULL) {
head = newNode;
} else {
struct Node* current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
```
4. 定义一个循环,用于遍历链表并输出每个节点的值:
```c
struct Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
```
完整的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
int main() {
// initialize the list with NULL
struct Node* head = NULL;
// insert values into the list
while (1) {
int value;
printf("Enter a value (-1 to exit): ");
scanf("%d", &value);
if (value == -1) {
break;
}
// create a new node
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = value;
newNode->next = NULL;
// insert the new node into the list
if (head == NULL) {
head = newNode;
} else {
struct Node* current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// print the values in the list
struct Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
// free the memory allocated for the list
current = head;
while (current != NULL) {
struct Node* temp = current;
current = current->next;
free(temp);
}
return 0;
}
```
阅读全文
相关推荐




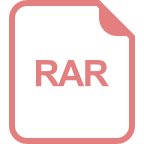











