为什么在上述的例子中能够保证 &hash_table的内存后面跟着的就是hash表的内容呢
时间: 2024-04-22 16:27:31 浏览: 76
在上面的例子中,我们使用了 `memcpy(shared_hash_table, &hash_table, sizeof(my_struct));` 将 `hash_table` 指针所指向的整个 `my_struct` 结构体(即整个 Hash 表结构体)复制到了共享内存区域中。由于 `hash_table` 是一个指向 `my_struct` 结构体的指针,其所指向的内存空间中包含了 `my_struct` 结构体中的所有字段。因此,在 `&hash_table` 内存后面跟着的就是 `my_struct` 结构体中的所有字段,也就是整个 Hash 表结构体中的所有键值对元素。因此,其他进程可以通过访问共享内存区域中的 `shared_hash_table` 指针,来访问整个 Hash 表结构体中的键值对元素。这样,我们就可以在多个进程之间共享同一个 Hash 表了。
相关问题
hash_table[3] = 0x8000; 该语句什么意思
这个语句 `hash_table[3] = 0x8000;` 的意思是将十六进制数 `0x8000` 存储到名为 `hash_table` 的哈希表中的索引为 3 的位置上。
哈希表是一种数据结构,可以将值与唯一的键关联起来。在这个例子中,`hash_table` 是一个数组或指针,其中每个索引位置都可以存储一个值。通过使用索引操作符 `[ ]`,我们可以访问和修改特定索引位置的值。
这个语句将 `0x8000` 存储到了 `hash_table` 的索引为 3 的位置上。`0x8000` 是一个十六进制数,表示为十进制数是 32768。因此,这个语句将在索引为 3 的位置上存储值 32768。
glibc hash_find 例子
glibc 中的 `hash_find` 函数用于在哈希表中查找指定键值对应的值。它的函数原型如下:
```c
void *hash_find (struct hash_table *table, const void *key)
```
其中,`table` 表示要查找的哈希表,`key` 是要查找的键值。
下面是一个 `hash_find` 函数的例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <search.h>
#define TABLESIZE 10
int main()
{
struct node {
char *name;
int age;
} *person;
struct node persons[] = {
{"Alice", 18},
{"Bob", 20},
{"Charlie", 22},
{"David", 24},
{"Emma", 26},
{"Frank", 28},
{"Grace", 30},
{"Henry", 32},
{"Ivy", 34},
{"John", 36}
};
struct hash_table *table;
table = (struct hash_table *) calloc(1, sizeof(struct hash_table));
if (table == NULL) {
perror("calloc");
exit(EXIT_FAILURE);
}
table->size = TABLESIZE;
table->hash = string_hash;
table->compare = strcmp;
hcreate(TABLESIZE);
int i;
for (i = 0; i < sizeof(persons) / sizeof(persons[0]); i++) {
person = &persons[i];
struct node **result = (struct node **) hsearch(table->entry, FIND, (void *) person->name, strlen(person->name));
if (result == NULL) {
perror("hsearch");
exit(EXIT_FAILURE);
} else if (*result != NULL) {
printf("%s already in table with age %d\n", (*result)->name, (*result)->age);
} else {
*result = person;
printf("%s added to table with age %d\n", person->name, person->age);
}
}
struct node **result = (struct node **) hsearch(table->entry, FIND, "Charlie", strlen("Charlie"));
if (result == NULL) {
perror("hsearch");
exit(EXIT_FAILURE);
} else if (*result == NULL) {
printf("Charlie not found in table\n");
} else {
printf("Charlie found in table with age %d\n", (*result)->age);
}
hdestroy();
return 0;
}
```
以上代码演示了如何使用 `hash_find` 函数在哈希表中查找指定键值对应的值。首先定义了一个 `node` 结构体表示一个人,包含了姓名和年龄两个属性。然后定义了一个 `persons` 数组,其中存储了一些人的信息。接着创建了一个 `hash_table` 结构体,并调用 `hcreate` 函数创建了一个哈希表。然后遍历 `persons` 数组,逐个将人的信息加入到哈希表中。在加入之前,先调用 `hsearch` 函数查找是否已经存在相同姓名的人。如果已经存在,就输出其年龄;否则,就将其加入到哈希表中。最后再次调用 `hsearch` 函数查找指定姓名的人的年龄,并输出其年龄。最后调用 `hdestroy` 函数销毁哈希表。
阅读全文
相关推荐







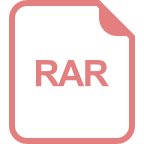

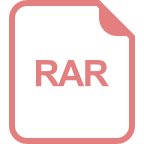






