设计实现一个简易版电商平台的代码。该电商平台要求使用Spring Cloud Alibaba微服务开发框架,且要使用学过的生态技术。
时间: 2024-02-16 12:59:58 浏览: 80
好的,我会给您提供一个简单的电商平台代码实现,使用Spring Cloud Alibaba微服务开发框架,以及一些学过的生态技术。由于篇幅限制,这里只展示部分代码片段。
首先,我们需要定义商品微服务的实体类和Repository接口:
```java
// 商品实体类
@Entity
@Table(name = "product")
@Data
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private Double price;
@Column(name = "create_time")
private Date createTime;
}
// 商品Repository接口
@Repository
public interface ProductRepository extends JpaRepository<Product, Long> {
}
```
然后,我们需要定义订单微服务的实体类和Repository接口:
```java
// 订单实体类
@Entity
@Table(name = "order")
@Data
public class Order {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private Long productId;
private Integer quantity;
private Double price;
@Column(name = "create_time")
private Date createTime;
}
// 订单Repository接口
@Repository
public interface OrderRepository extends JpaRepository<Order, Long> {
}
```
接下来,我们可以使用Feign来实现商品微服务和订单微服务之间的调用:
```java
// 商品微服务的Feign接口
@FeignClient(name = "product-service")
public interface ProductFeignClient {
@GetMapping("/products/{id}")
Product getProductById(@PathVariable("id") Long id);
}
// 订单微服务的Feign接口
@FeignClient(name = "order-service")
public interface OrderFeignClient {
@PostMapping("/orders")
Order createOrder(@RequestBody Order order);
}
```
然后,我们可以使用RabbitMQ来实现订单微服务的消息处理:
```java
// 订单微服务的消息处理器
@Service
public class OrderMessageHandler {
@Autowired
private OrderRepository orderRepository;
@RabbitListener(queues = "order-queue")
public void handleMessage(Order order) {
orderRepository.save(order);
}
}
```
最后,我们可以使用Vue.js等前端框架来实现用户界面,如下所示:
```vue
<template>
<div>
<h1>商品列表</h1>
<ul>
<li v-for="product in products" :key="product.id">
<span>{{ product.name }}</span>
<span>{{ product.price }}</span>
<button @click="createOrder(product.id, 1, product.price)">购买</button>
</li>
</ul>
</div>
</template>
<script>
import { ProductFeignClient, OrderFeignClient } from '@/api'
export default {
data () {
return {
products: []
}
},
created () {
ProductFeignClient.getProducts().then(res => {
this.products = res.data
})
},
methods: {
createOrder (productId, quantity, price) {
const order = {
productId,
quantity,
price
}
OrderFeignClient.createOrder(order).then(res => {
console.log('订单创建成功')
})
}
}
}
</script>
```
以上就是一个简单的电商平台代码实现,当然实际操作中还需要根据具体情况进行调整和完善。
阅读全文
相关推荐
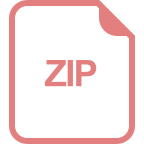
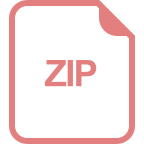

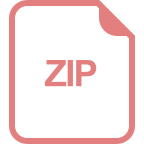
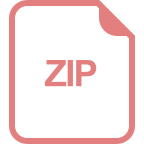
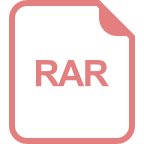
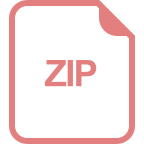
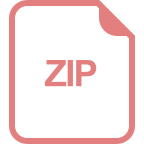
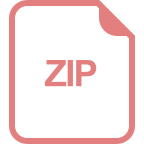
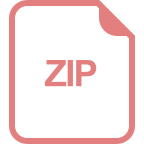
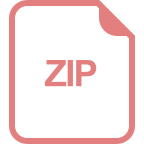
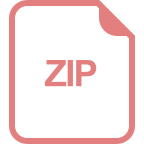
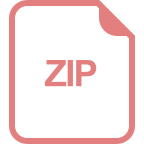
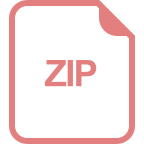
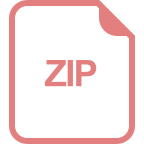
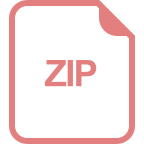
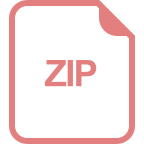