用python写出其中5个函数/类的使用示例代码。1:len函数 ## 2:zip函数 ## 3:map函数 ## 4:sorted函数 ## 5:collections.Counter类
时间: 2024-12-17 19:18:35 浏览: 1
### 1. len 函数:
`len()` 函数用于返回序列、集合或其他可迭代对象的长度。例如:
```python
str_example = "Hello, World!"
print(len(str_example)) # 输出:13
list_example = [1, 2, 3, 4, 5]
print(len(list_example)) # 输出:5
```
### 2. zip 函数:
`zip()` 可以同时遍历多个列表,将元素配对成元组,返回的是一个迭代器。
```python
names = ["Alice", "Bob"]
ages = [20, 25]
for name, age in zip(names, ages):
print(f"{name} is {age} years old.") # 输出:Alice is 20 years old., Bob is 25 years old.
```
### 3. map 函数:
`map()` 函数对序列中的每个元素应用给定的函数,返回一个新的迭代器。
```python
def square(x): return x**2
numbers = [1, 2, 3, 4, 5]
squared_numbers = map(square, numbers)
print(list(squared_numbers)) # 输出:[1, 4, 9, 16, 25]
```
### 4. sorted 函数:
`sorted()` 对可迭代对象排序,返回一个新的已排序列表。
```python
unsorted_list = [3, 1, 4, 1, 5, 9]
sorted_list = sorted(unsorted_list)
print(sorted_list) # 输出:[1, 1, 3, 4, 5, 9]
```
### 5. collections.Counter 类:
`collections.Counter` 记录每个元素出现的次数,主要用于统计频率。
```python
from collections import Counter
word_list = ['apple', 'banana', 'apple', 'cherry', 'banana', 'banana']
counter = Counter(word_list)
print(counter) # 输出:Counter({'banana': 3, 'apple': 2, 'cherry': 1})
```
阅读全文
相关推荐
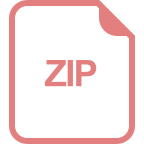
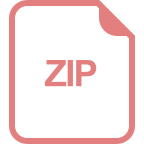
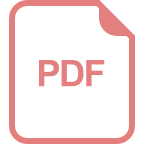















